Lazy Programming Series – External & Built-in Modules | F-Strings & string format in Python
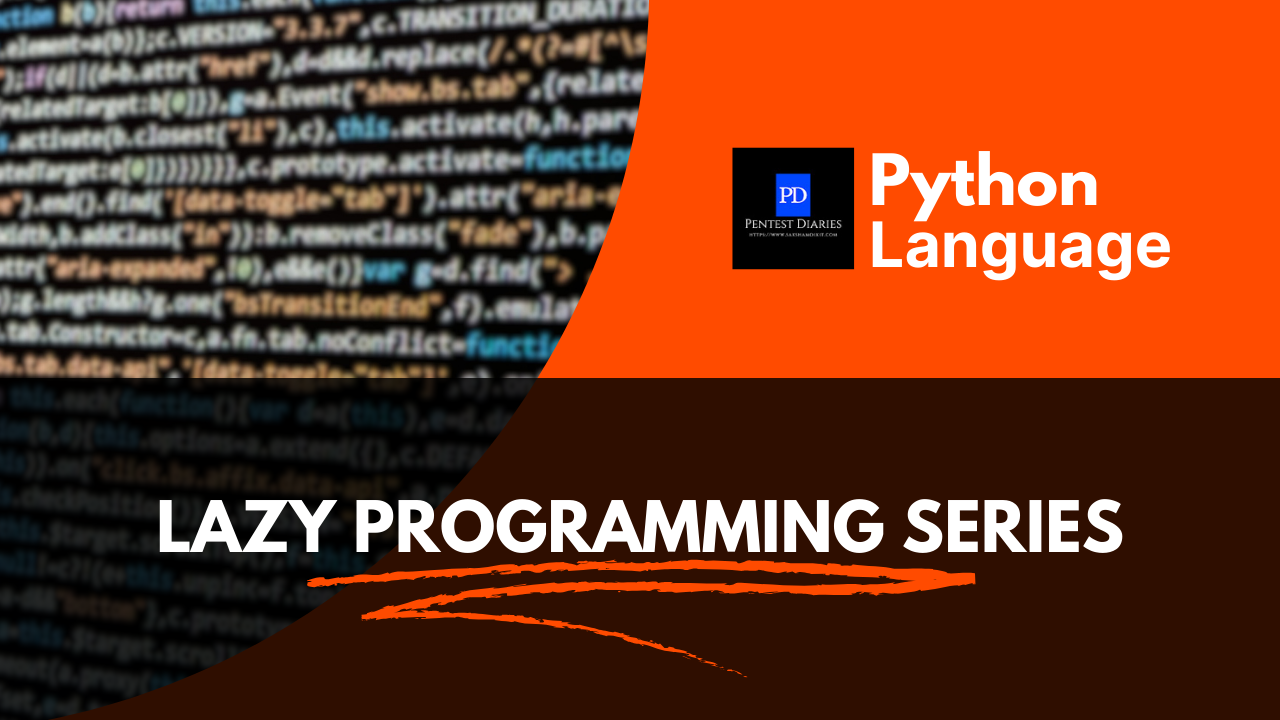
External & Built-in Modules in Python
Python provides a rich ecosystem of both built-in modules and external libraries (also known as packages) that you can use to enhance your programs. Here’s a brief overview of both:
Built-in Modules:
Python comes with a set of modules that are available out of the box without the need for installing anything extra. These include modules for common tasks like working with files, handling dates and times, performing mathematical operations, and more. Some examples of built-in modules are:
- os: Provides a way to interact with the operating system, including file operations, directory operations, etc.
- datetime: Allows manipulation of dates and times.
- math: Provides mathematical functions.
- random: Enables generation of random numbers.
- json: Allows encoding and decoding JSON data.
External Modules:
In addition to the built-in modules, Python has a vast ecosystem of external libraries that you can install using tools like pip (Python’s package manager). These libraries cover a wide range of functionalities and can be easily integrated into your projects. Some popular external libraries include:
- numpy: A powerful library for numerical computing, especially for working with arrays and matrices.
- pandas: A data manipulation and analysis library, useful for working with structured data.
- matplotlib and seaborn: Libraries for creating data visualizations and plots.
- requests: A library for making HTTP requests, useful for interacting with web APIs.
- tensorflow and pytorch: Libraries for machine learning and deep learning.
To use external modules, you typically need to install them first using pip. For example, to install the requests module, you would run:

Once installed, you can import and use these modules in your Python scripts just like you would with built-in modules:
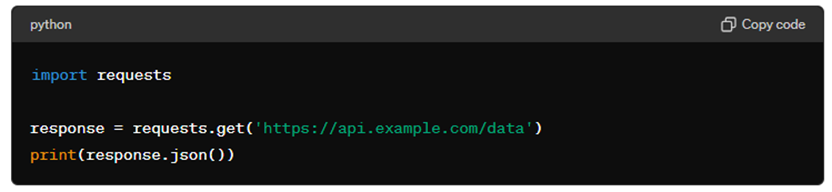
By leveraging both built-in and external modules, you can extend Python’s capabilities to suit your specific needs and build powerful applications.
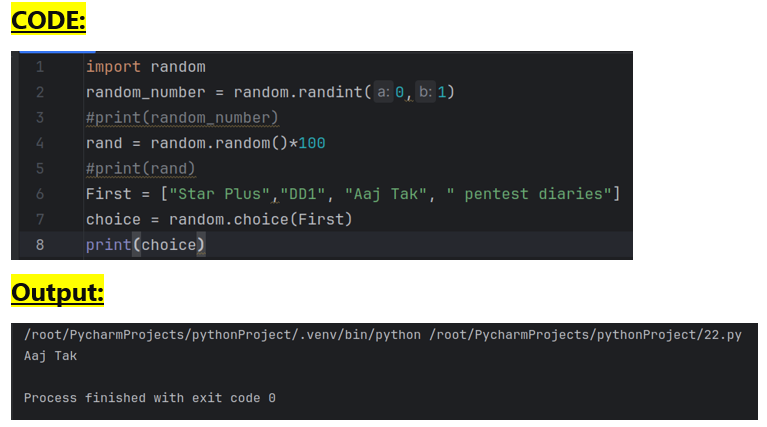
F-Strings & string format in Python
F-strings and string formatting are powerful tools in Python for creating formatted strings. Here’s an overview of both:
F-Strings (Formatted String Literals):
Introduced in Python 3.6, f-strings provide a concise and readable way to embed expressions inside string literals. You can use f-strings by prefixing a string literal with f
or F
and then including expressions within curly braces {}
. These expressions are evaluated at runtime and their values are inserted into the string.
Example:
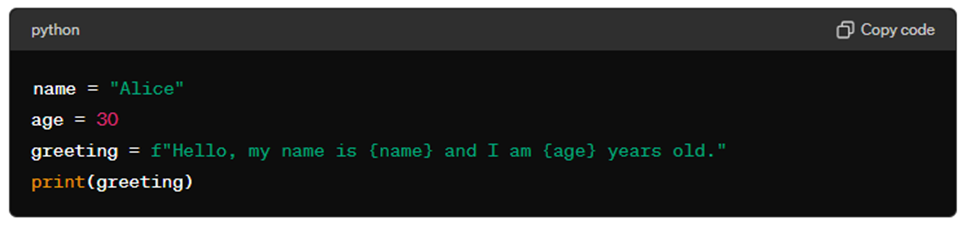
Output:

F-strings support various formatting options such as specifying precision for floating-point numbers, padding strings, and more.
String Formatting with str.format()
:
Before the introduction of f-strings, str.format()
was the preferred method for string formatting in Python. With str.format()
, you create a string template with placeholder {}
for the values you want to insert. You then call the format()
method on the string and pass the values to be inserted as arguments.
Example:
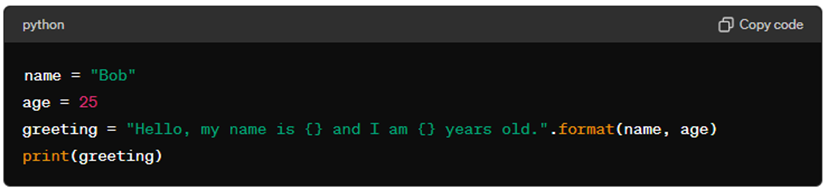
Output:

You can also specify the position of the arguments or use keyword arguments for more clarity and flexibility.
Example:

Output:

Both f-strings and str.format()
offer similar functionality, but f-strings are often preferred for their readability and simplicity, especially when working with Python 3.6 and later versions. However, str.format()
remains useful in cases where you need more control over formatting or when working with older versions of Python.
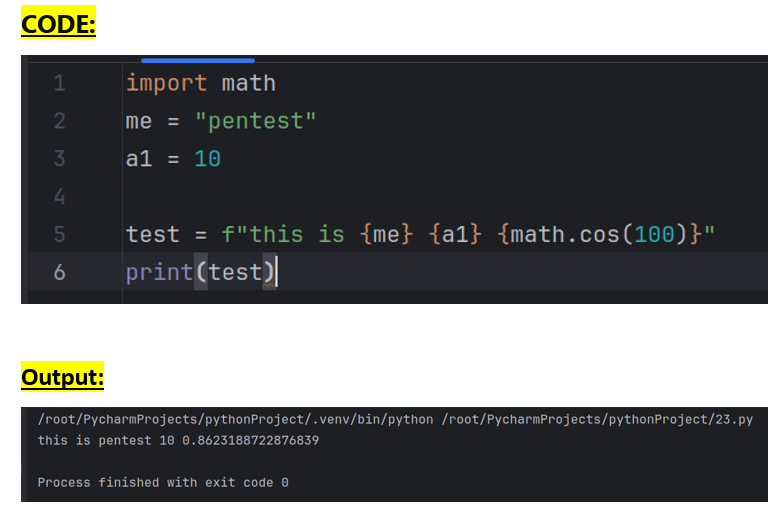