Lazy Programming Series – TIME Module, VIRTUAL ENVIRONMENT & REQUIREMENT & Enumerate Function in Python
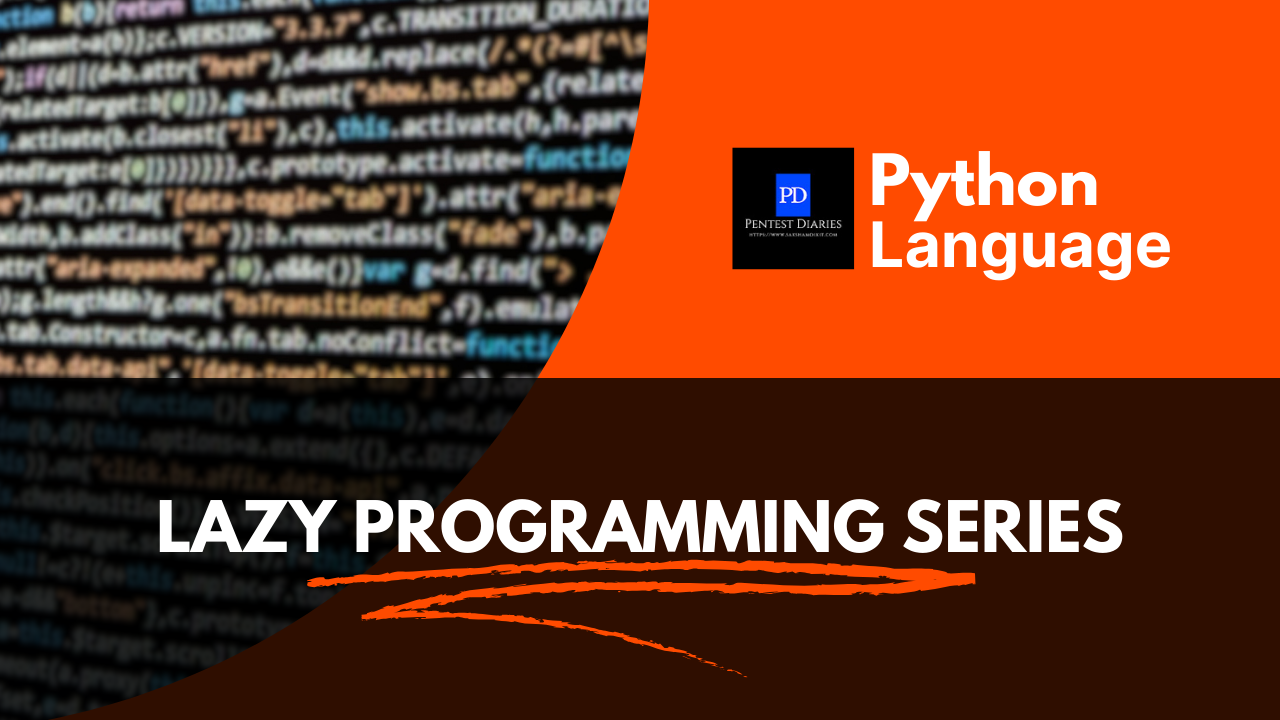
TIME Module in Python:
The time module in Python provides various functions to work with time-related tasks, such as getting the current time, pausing execution, converting between different time representations, and measuring elapsed time. Here’s an overview of some commonly used functions and constants in the time module:
Getting Current Time:
- time(): Returns the current time in seconds since the epoch (January 1, 1970, 00:00:00 UTC) as a floating-point number.

Converting Time:
- ctime(): Converts a timestamp (seconds since the epoch) to a human-readable string representation of the local time.

Pausing Execution:
- sleep(seconds): Suspends execution of the current thread for the given number of seconds.
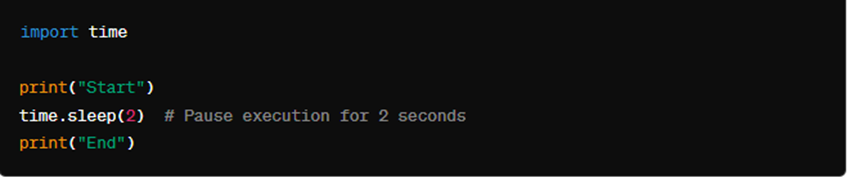
Formatting Time:
- strftime(format, time_tuple): Converts a time tuple or a struct_time object to a string representation based on the format specified.
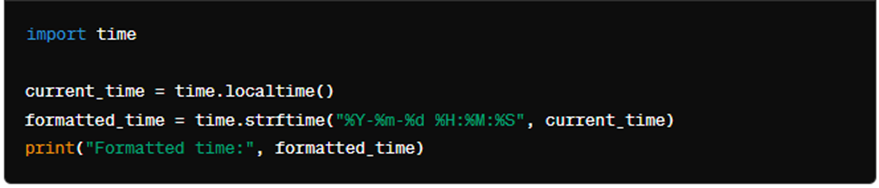
Measure Elapsed Time:
- perf_counter(): Returns a high-resolution time value (in fractional seconds) intended for use in measuring short durations.
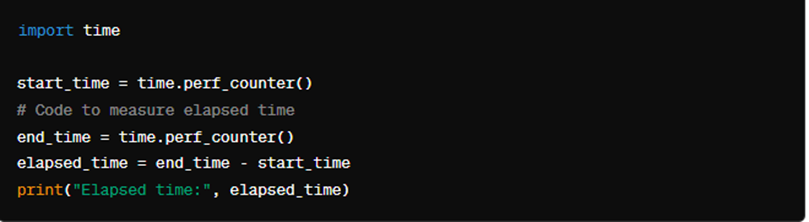
Constants:
- time.localtime(): Returns the current time as a struct_time object, representing the local time.
- time.gmtime(): Returns the current time as a struct_time object, representing the UTC time.
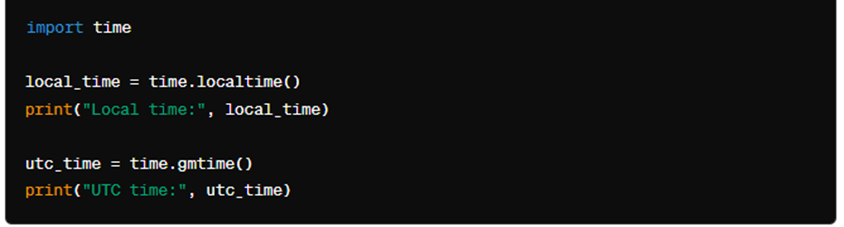
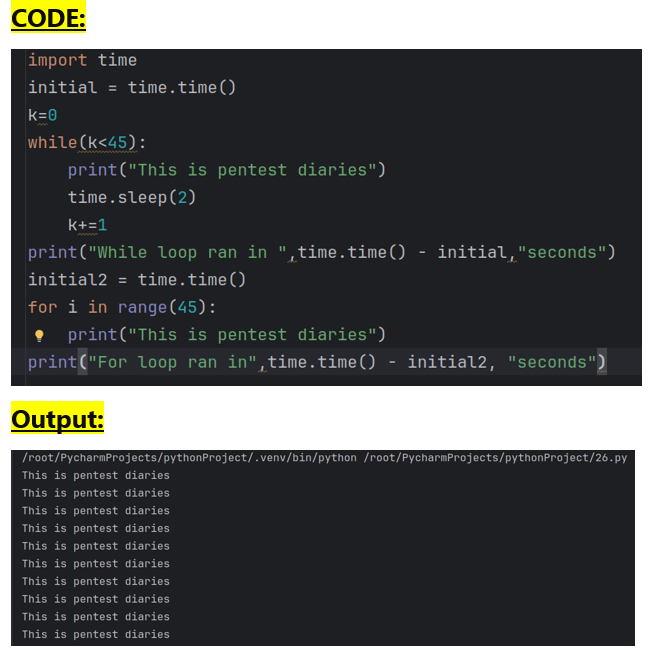
VIRTUAL ENVIRONMENT & REQUIREMENT:
In Python, virtual environments and requirements files are essential tools for managing project dependencies and isolating project environments. Here’s a brief overview of each:
- Virtual Environments:
A virtual environment is a self-contained directory tree that contains a Python installation for a particular version of Python, as well as a number of additional packages. It allows you to work on a Python project without affecting the system-wide Python installation or other projects.
To create a virtual environment, you can use the built-in venv
module in Python 3:

This command creates a new virtual environment named myenv
. You can activate it by running:
On Windows:

On Unix or MacOS:

- Once activated, any Python commands you run will use the Python interpreter and packages installed within the virtual environment.
- Requirements Files:
A requirements file is a simple text file that lists all the Python packages that your project depends on, along with their versions. This file allows you to specify exactly which packages and versions are required for your project, making it easier to reproduce the environment on another machine.
You can generate a requirements file that captures the current state of your virtual environment using the pip freeze
command:

This will create a file named requirements.txt
containing a list of all installed packages and their versions. You can then share this file with others, and they can use it to install the exact same set of dependencies by running:

This ensures that everyone working on the project is using the same versions of the dependencies, reducing the chances of compatibility issues.
By combining virtual environments with requirements files, you can create isolated and reproducible development environments for your Python projects.
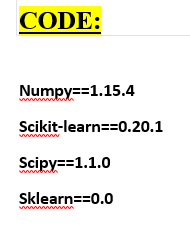
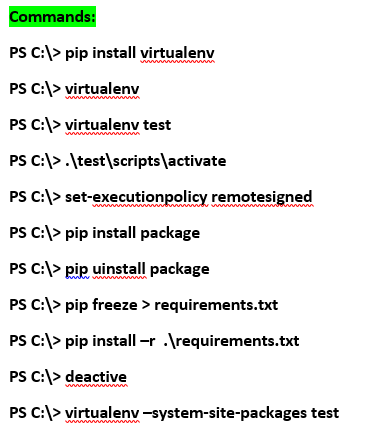
ENUMERATE FUNCTION:
The enumerate() function in Python is a built-in function that allows you to loop over an iterable (such as a list, tuple, or string) while also keeping track of the index of the current item. It returns an enumerate object, which yields pairs of indices and corresponding values from the iterable.
Here’s the syntax of the enumerate() function:

- iterable: The iterable (list, tuple, string, etc.) that you want to loop over.
- start (optional): The index at which to start counting. By default, it is 0.
Here’s a simple example to demonstrate how enumerate() works:
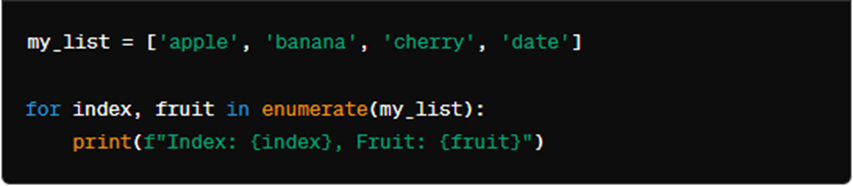
Output:
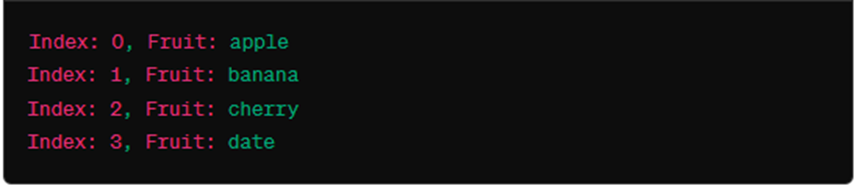
In this example, the enumerate() function is used to iterate over the my_list list. In each iteration, it returns a tuple containing the index of the current item and the item itself. This tuple is then unpacked into the variables index and fruit, which are then used in the loop body.
You can also specify a starting index other than 0 if needed:

Output:
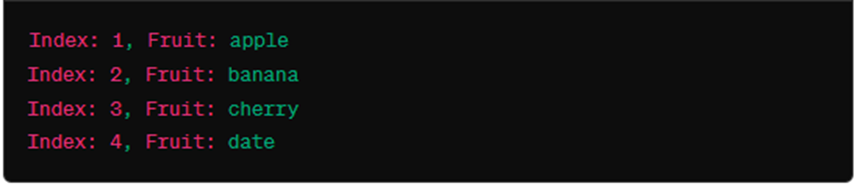
In this case, the enumeration starts from 1 instead of the default 0.
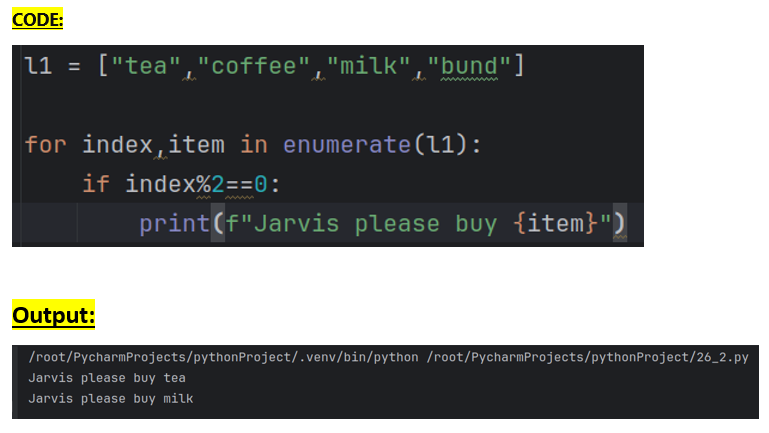
@SAKSHAM DIXIT