Lazy Programming Series – Join Function & (Map, Filter & Reduce) in Python
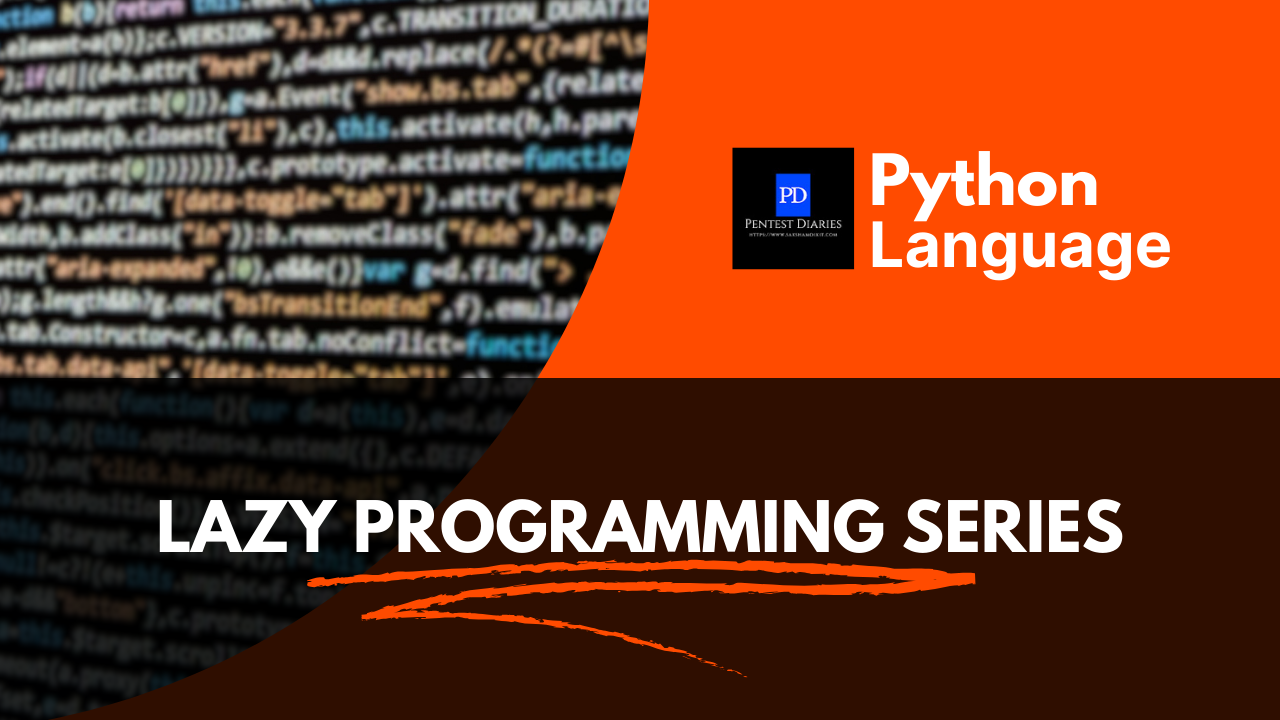
Join Function in Python:
In Python, the join function is used to concatenate the elements of an iterable (like a list or tuple) into a single string, with a specified string (the separator) in between each element. This method is particularly useful for creating a single string from a list of strings.
Syntax:

separator
: The string that you want to use as the separator between the elements.iterable
: The iterable (like a list or tuple) whose elements you want to join into a single string.
Examples:

Output:

Using a Comma as a Separator

Output:

Joining with No Separator.

Output:

Edge Cases
- If the iterable contains non-string elements, a
TypeError
will be raised. Ensure all elements are strings before usingjoin
. - If the iterable is empty, the result will be an empty string.
Example with Error Handling
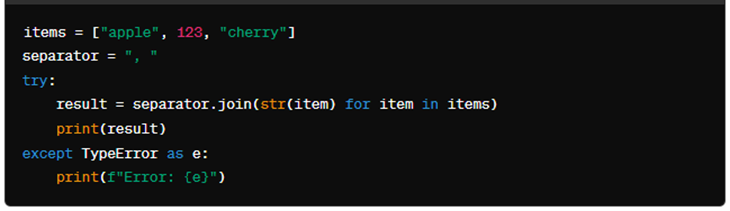
Output:

In this example, we convert each item to a string using a generator expression before joining them, which avoids the TypeError
.
Using the join
function effectively can make your code cleaner and more readable, especially when dealing with string concatenation.
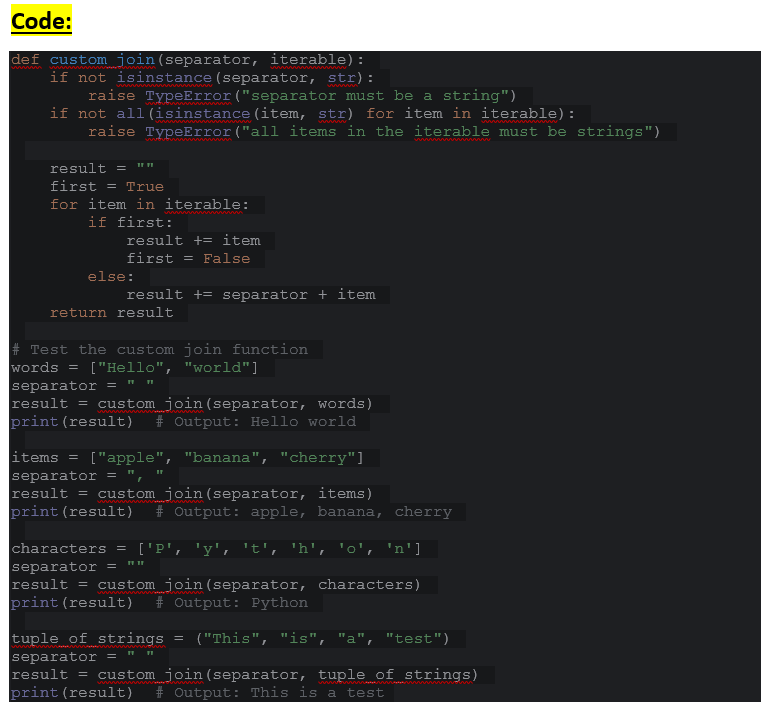

MAP, Filter & Reduce:
In Python, map
, filter
, and reduce
are functional programming tools that allow you to apply functions to sequences in a concise and expressive way. Here’s a brief overview of each:
1. map()
The map()
function applies a given function to all items in an input list (or any iterable) and returns a map object (which is an iterator).
Syntax:

Example:
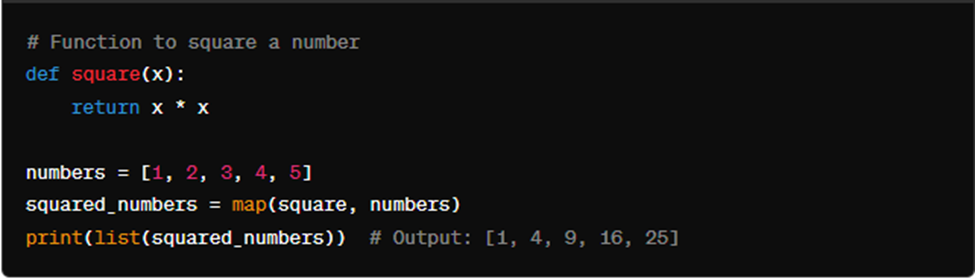
Using a lambda function with map
:

2. filter()
The filter()
function constructs an iterator from elements of an iterable for which a function returns true.
Syntax:

Example:
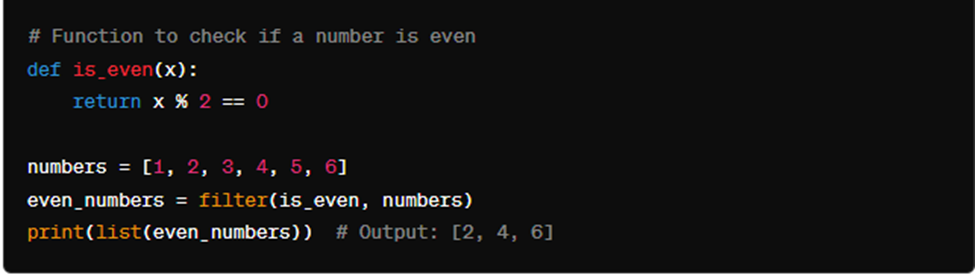
Using a lambda function with filter
:

3. reduce()
The reduce()
function from the functools
module applies a rolling computation to sequential pairs of values in an iterable and reduces it to a single value.
Syntax:

Example:
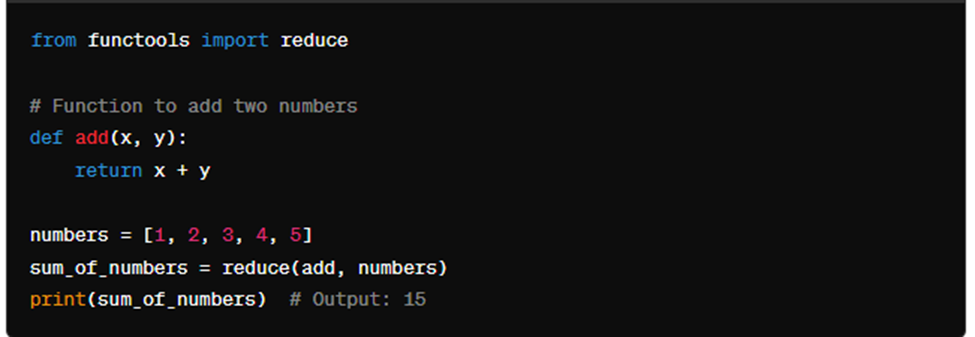
Using a lambda function with reduce
:
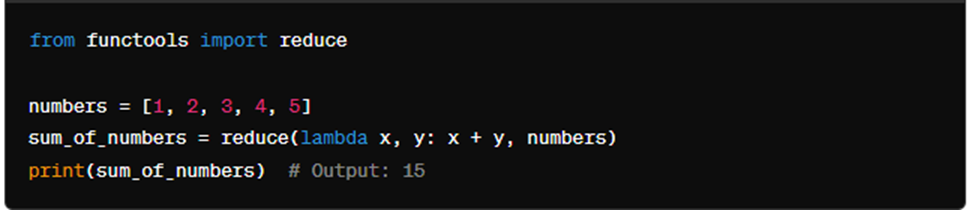
Summary
- map(): Applies a function to all items in an iterable.
- filter(): Filters items in an iterable based on a function that returns a boolean value.
- reduce(): Applies a function cumulatively to the items in an iterable, reducing it to a single value.
These functions enable a functional programming approach in Python, making code more readable and expressive when dealing with transformations and reductions on iterables.

from functools import reduce
# Sample data
numbers = [1, 2, 3, 4, 5]
# 1. Map: Apply a function to each element in the list.
# Let’s square each number in the list.
squared_numbers = list(map(lambda x: x ** 2, numbers))
print(f”Squared Numbers: {squared_numbers}”)
# 2. Filter: Filter elements that meet a condition.
# Let’s filter out even numbers.
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(f”Even Numbers: {even_numbers}”)
# 3. Reduce: Apply a function cumulatively to the items of a sequence.
# Let’s calculate the product of all numbers.
product = reduce(lambda x, y: x * y, numbers)
print(f”Product of Numbers: {product}”)

Explanation
Map: The map
function applies the given lambda function (lambda x: x ** 2
) to each element in the numbers
list, resulting in a new list of squared numbers.

Filter: The filter
function applies the given lambda function (lambda x: x % 2 == 0
) to each element in the numbers
list and returns a new list containing only the elements that satisfy the condition (even numbers).

Reduce: The reduce
function applies the given lambda function (lambda x, y: x * y
) cumulatively to the items of the numbers
list, from left to right, to reduce the list to a single value (the product of all numbers).

When you run this code, you will get the following output:

This example demonstrates how to use map
, filter
, and reduce
to process and transform lists in Python.
@SAKSHAM DIXIT