Lazy Programming Series – Instance and Class variables in python
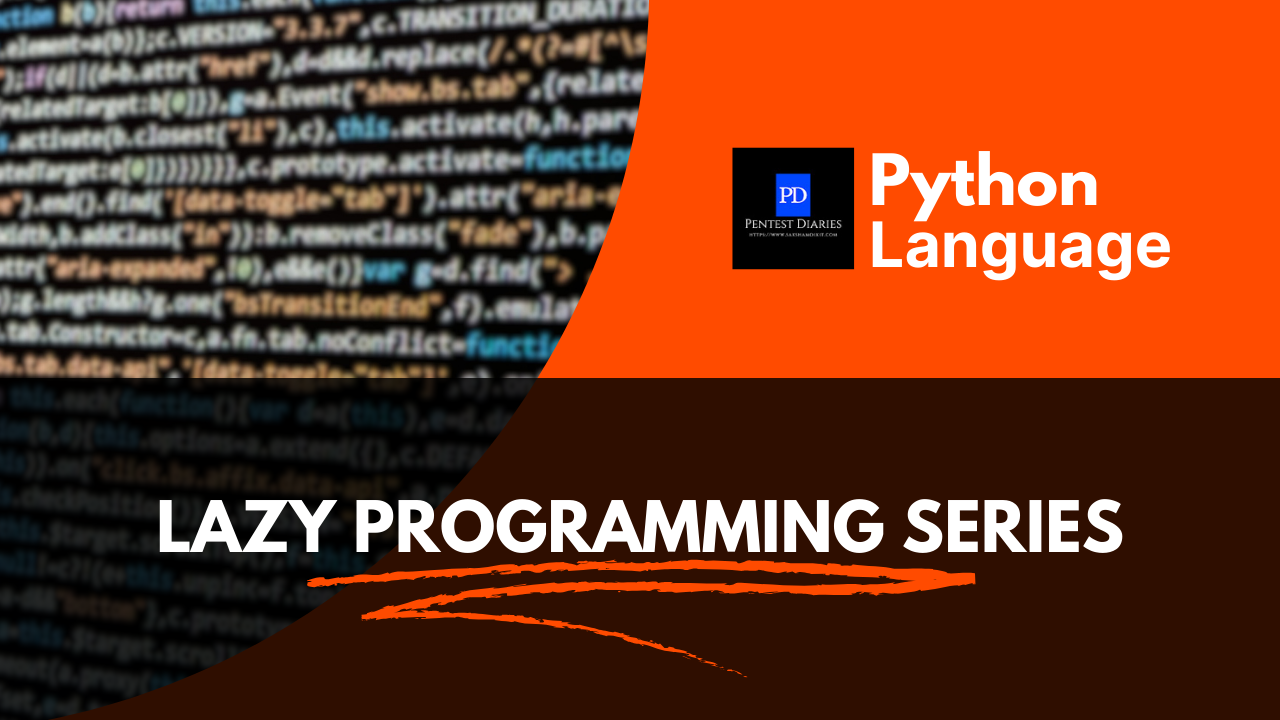
Instance and Class variables in Python
In Python, classes can have two types of variables: instance variables and class variables. Understanding the difference between these two is crucial for managing the state and behavior of your objects properly.
Instance Variables
Instance variables are attributes that are specific to each instance of a class. They are defined within methods (typically the __init__ method) and are prefixed with self to denote that they belong to the instance.
Defining Instance Variables
Instance variables are usually initialized inside the __init__ method, which is the constructor for the class. Each instance of the class will have its own copy of these variables.
Example:
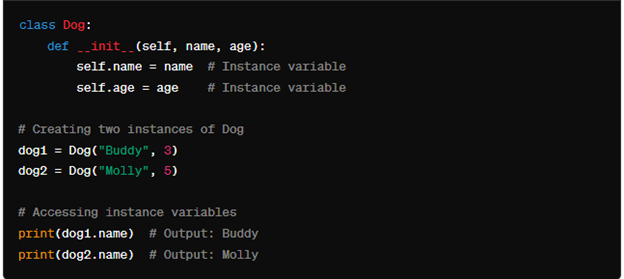
In this example, name
and age
are instance variables. dog1
and dog2
have their own separate name
and age
attributes.
Class Variables
Class variables are attributes that are shared by all instances of a class. They are defined within the class but outside any methods. All instances of the class share the same value for class variables.
Defining Class Variables
Class variables are typically defined directly beneath the class definition and outside of any instance methods.
Example:
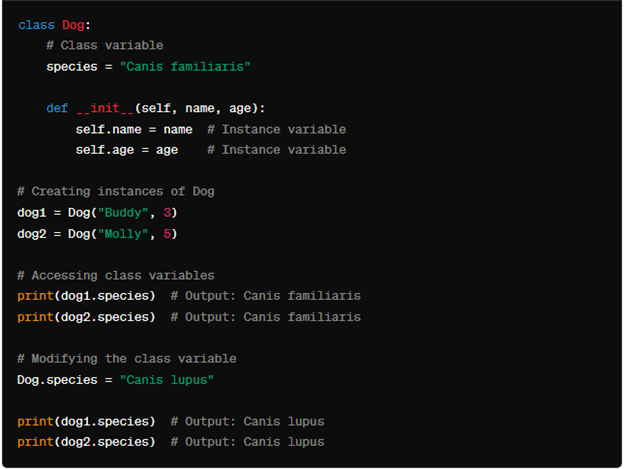
In this example, species
is a class variable. Changing Dog.species
affects all instances of the class.
Differences Between Instance and Class Variables
- Scope:
- Instance Variables: Scoped to the instance; each instance has its own copy.
- Class Variables: Scoped to the class; shared across all instances.
- Initialization:
- Instance Variables: Initialized inside methods (usually
__init__
) withself
. - Class Variables: Initialized directly within the class definition.
- Access:
- Instance Variables: Accessed and modified through
self
. - Class Variables: Accessed through the class name or instances, but typically modified through the class name.
Example: Combining Instance and Class Variables
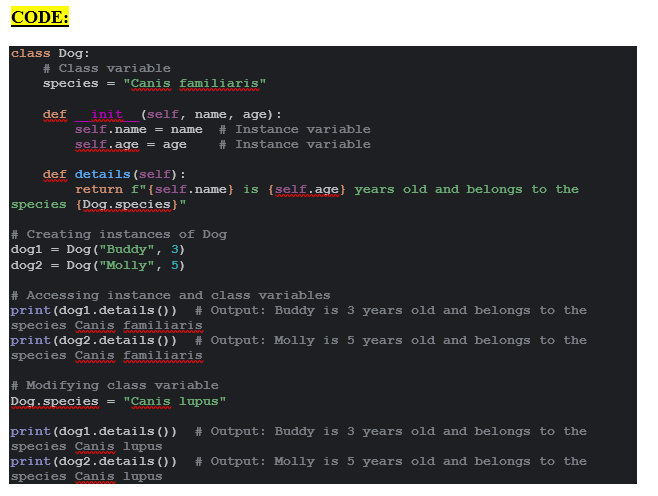
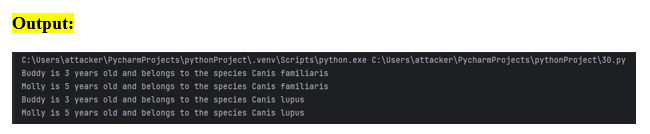
Summary
- Instance Variables: Specific to each instance, defined within methods using self.
- Class Variables: Shared among all instances, defined directly within the class.
- Use instance variables for data unique to each instance.
- Use class variables for data shared across all instances.
Understanding these concepts helps in designing classes that maintain proper state and behavior, providing a clear structure to your code.
@SAKSHAM DIXIT