Lazy Programming Series – Advanced List Slicing, Bisect Module, Format & Join Function, Is vs == in Python
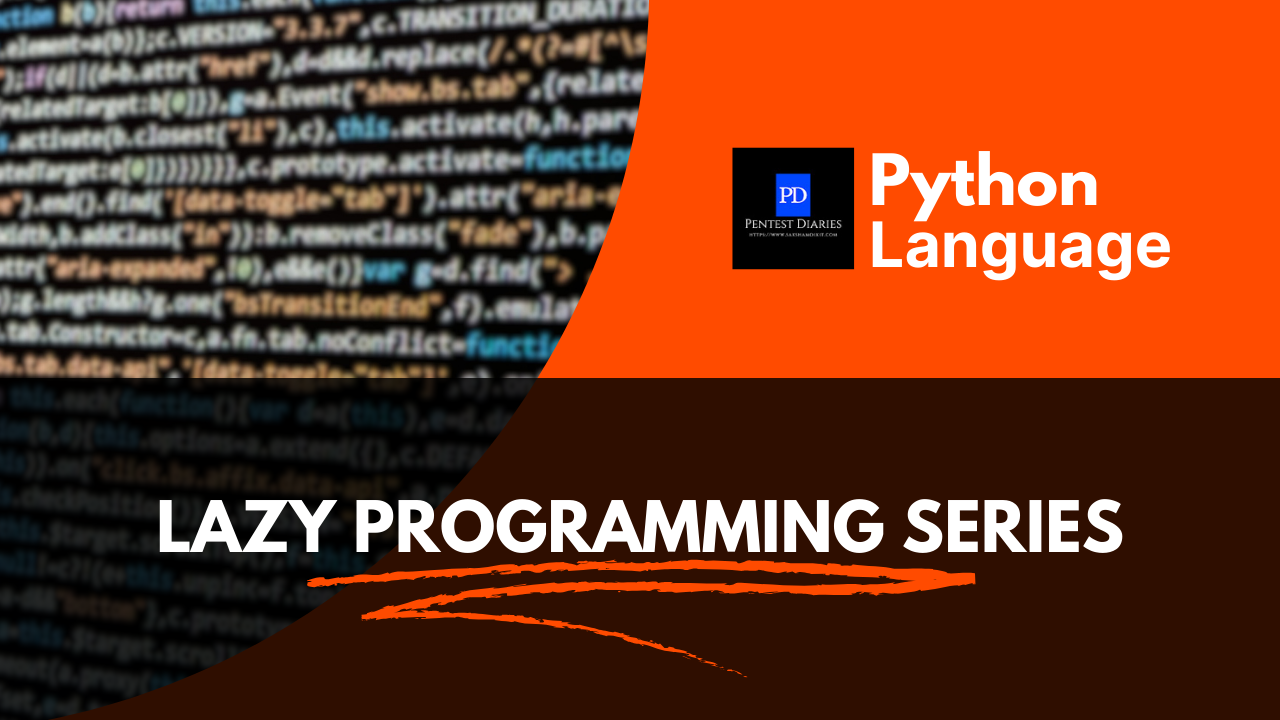
Advanced List Slicing in Python
List slicing in Python provides a powerful mechanism for extracting elements from a list based on their positions. Advanced list slicing takes this concept further, enabling you to extract sublists with more complex patterns or criteria.
Basic List Slicing
Before going into advanced slicing, let’s review basic list slicing:
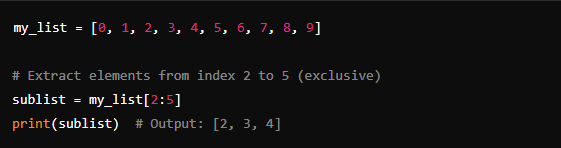
Advanced List Slicing Techniques
Step Size: You can specify a step size to skip elements while slicing.

Negative Indices: Negative indices count from the end of the list.
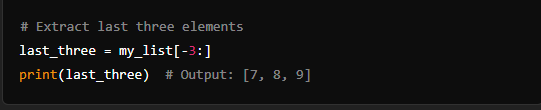
Reverse a List: Use a negative step size to reverse the list.

Skipping Elements with Step Size: You can combine step size with negative indices.

Conditional Slicing: Use a conditional expression inside square brackets to filter elements based on a condition.

Slicing with Functions: You can use functions to filter elements dynamically.
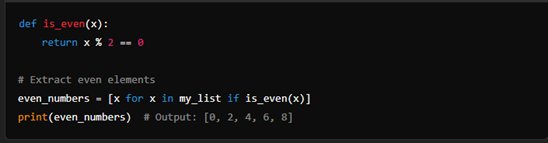
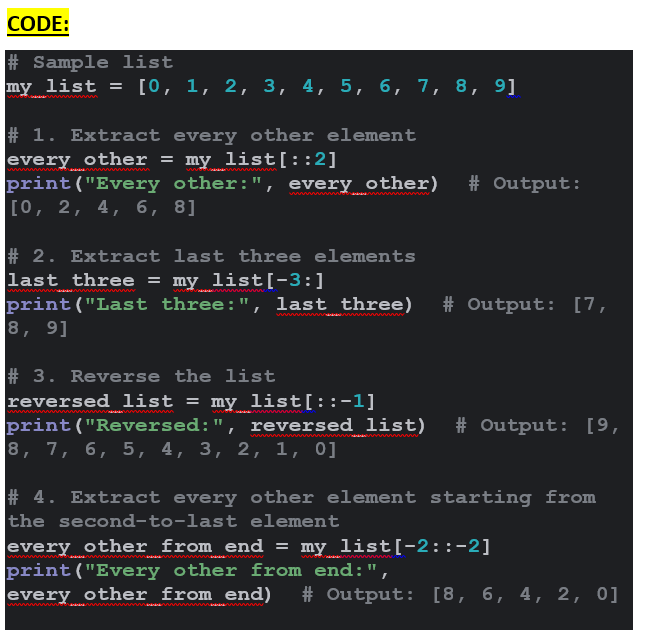
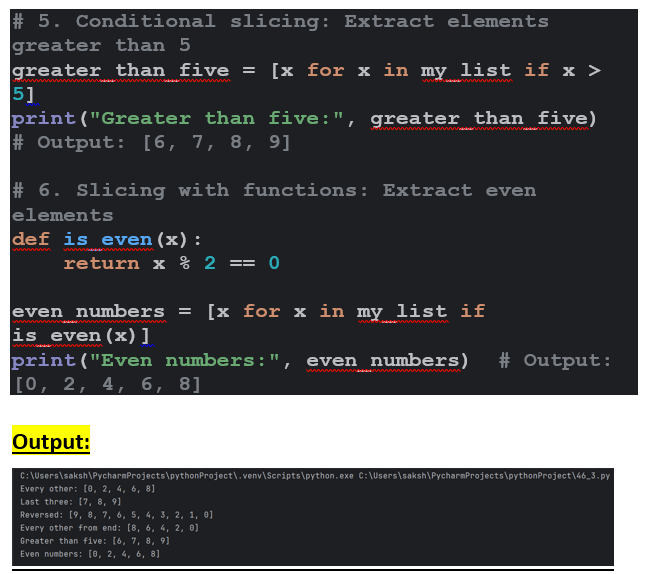
BISECT MODULE in Python:
The bisect
module in Python provides support for maintaining a list in sorted order without having to explicitly sort the list after each insertion. It achieves this through efficient binary search algorithms for inserting and locating elements in a sorted sequence.
Basic Functionality
The bisect
module primarily includes two main functions: bisect_left()
and bisect_right()
. These functions find the insertion points for a specified element in a sorted list to maintain its sorted order.
bisect_left(a, x, lo=0, hi=len(a))
: This function finds the index where the elementx
should be inserted in the sorted lista
to maintain its sorted order. If the element is already present, it returns the leftmost insertion point. Thelo
andhi
parameters allow specifying a subrange of the list to search within.bisect_right(a, x, lo=0, hi=len(a))
: Similar tobisect_left()
, this function finds the rightmost insertion point for the elementx
in the sorted lista
.
Example Usage
Let’s explore how you can use the bisect
module in practice:
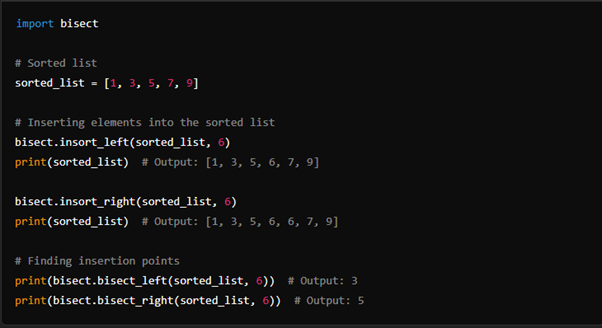
In this example:
- We start with a sorted list
[1, 3, 5, 7, 9]
. - We use
insort_left()
andinsort_right()
to insert the element6
into the list while maintaining its sorted order. - We use
bisect_left()
andbisect_right()
to find the leftmost and rightmost insertion points for the element6
in the list.
Additional Functions
The bisect
module also provides other functions like bisect()
and insort()
which are aliases for bisect_right()
and insort_right()
respectively. These functions are more intuitive for many users as they return the same insertion point as bisect_right()
and insert elements in the same way as insort_right()
.
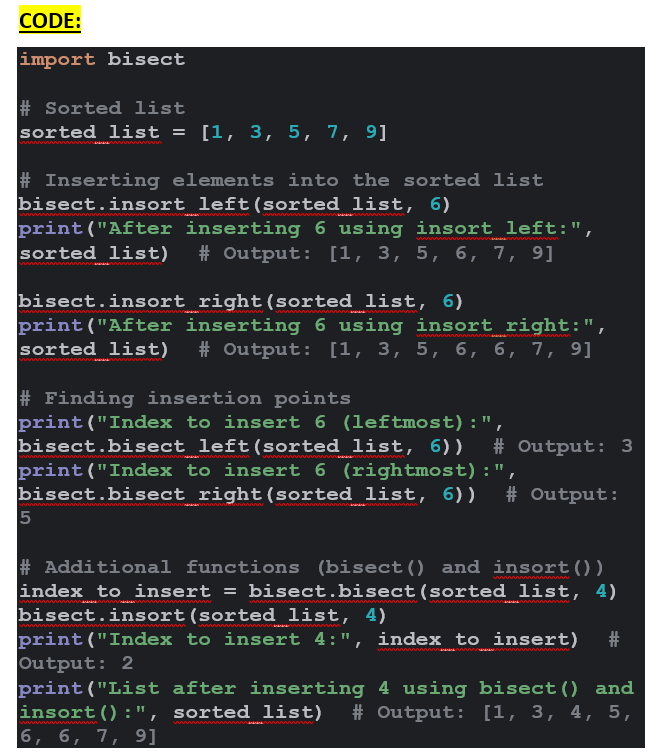
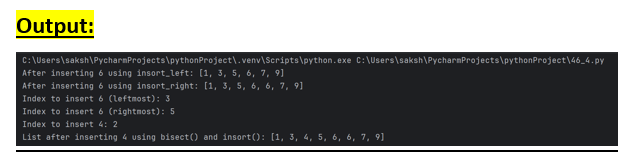
FORMAT Function in Python:
The format()
function in Python is a powerful method for formatting strings. It allows you to create dynamic strings with placeholders that can be replaced by values or variables. This function provides flexibility and control over how data is presented in your Python programs.
Basic Syntax
The basic syntax of the format()
function is as follows:

Here, template_string
is a string containing placeholders (curly braces {}
), and value1
, value2
, etc., are the values or variables used to replace the placeholders.
Positional and Keyword Arguments
You can pass arguments to the format()
function as positional or keyword arguments. Positional arguments are replaced in the order they appear, while keyword arguments are replaced based on the keys they correspond to in the template string.
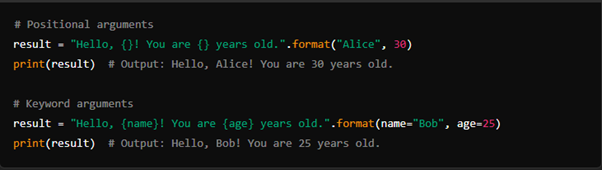
Formatting Options
The format()
function supports various formatting options to control how values are displayed, including:
- Specifying the number of decimal places for floating-point numbers.
- Controlling the alignment and width of strings.
Adding leading zeros to integers.
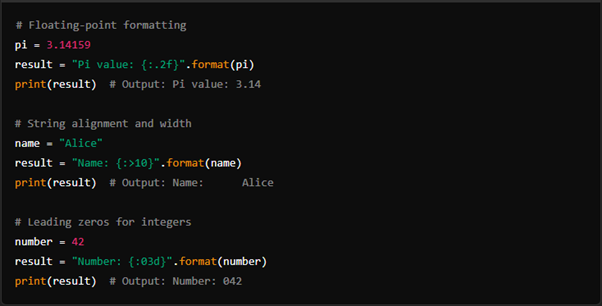
Advanced Usage
The format()
function also allows for more advanced formatting, including using index-based placeholders, accessing attributes of objects, and calling methods on objects.
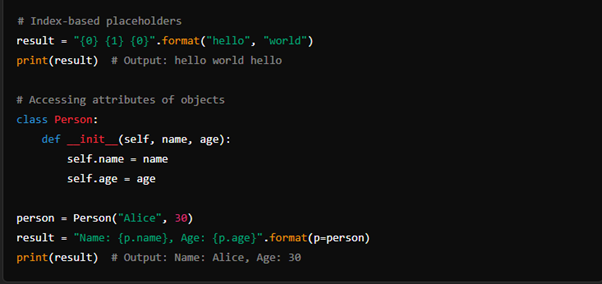
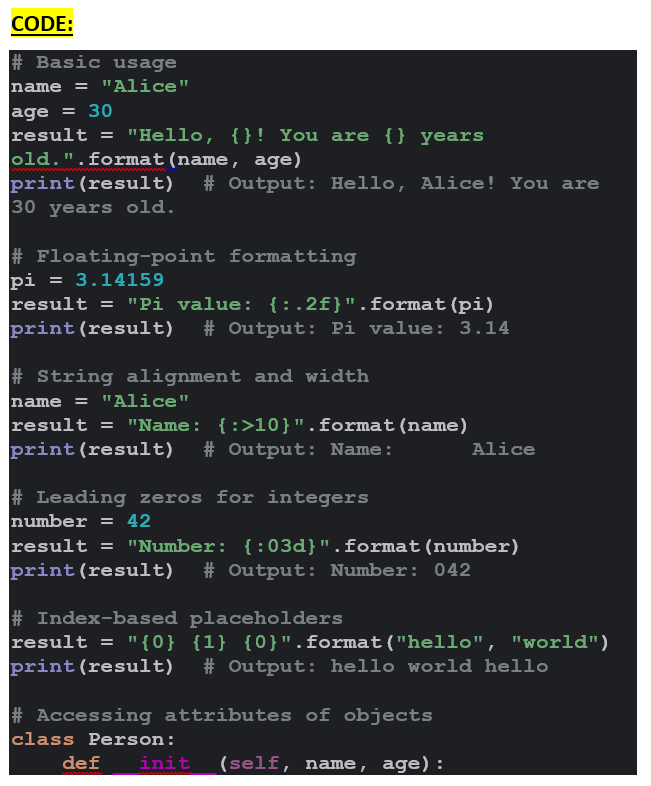
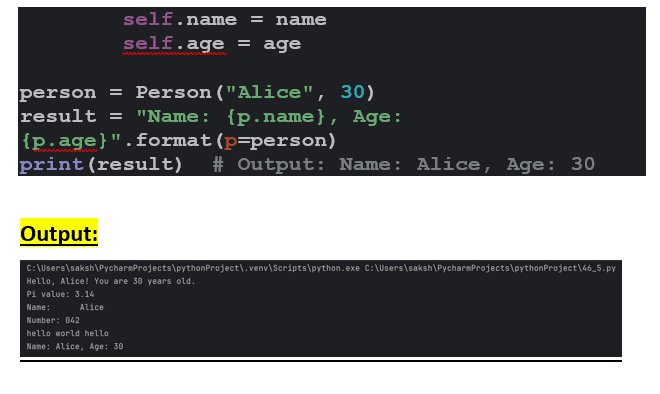
JOIN Function in Python
The join()
function in Python is a powerful method for joining the elements of an iterable (such as a list, tuple, or string) into a single string. It is particularly useful when you need to concatenate multiple strings together efficiently.
Basic Syntax
The basic syntax of the join()
function is as follows:

Here, separator_string
is the string that will be used to join the elements, and iterable
is the iterable containing the elements to be joined.
Example Usage
Let’s explore how you can use the join()
function in practice:
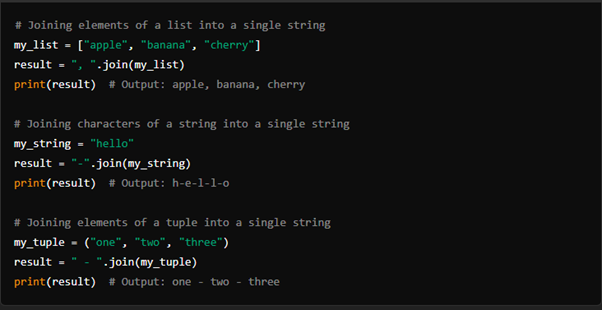
Handling Different Types of Iterables
The join()
function can work with various types of iterables, including lists, tuples, and strings. However, it expects all elements of the iterable to be strings. If the iterable contains non-string elements, you’ll need to convert them to strings first.

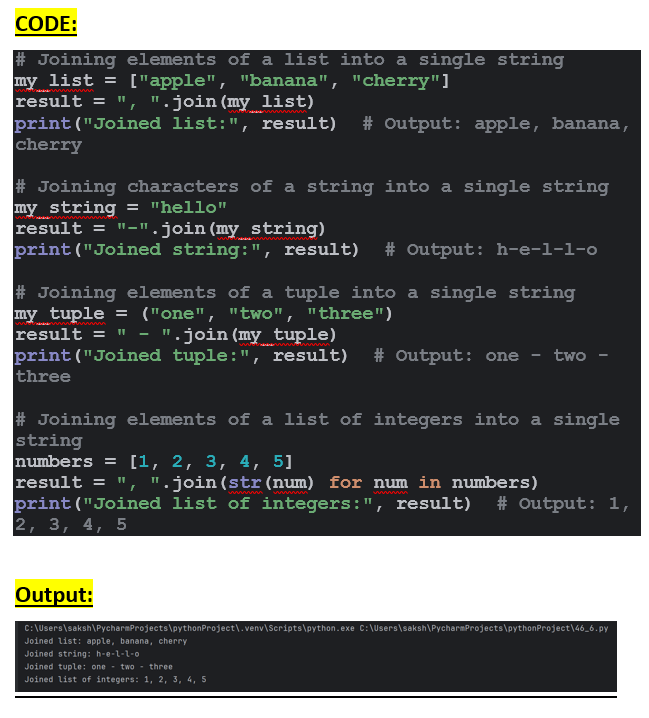
‘is” vs ‘==’ & what is the difference in python:
In Python, is
and ==
are two different operators used for comparison, and they have different behaviors:
is
Operator:
The is
operator checks whether two variables refer to the same object in memory, i.e., it checks if the two variables are pointing to the same memory location.
==
Operator:
The ==
operator checks whether the values of two variables are equal or not, i.e., it compares the values stored in the variables regardless of whether they refer to the same object in memory.
Here’s a code example to illustrate the difference:
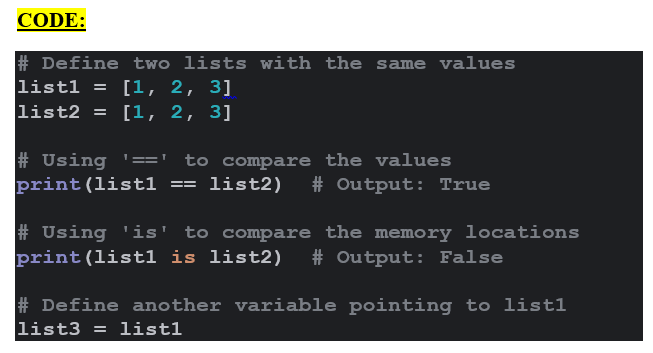
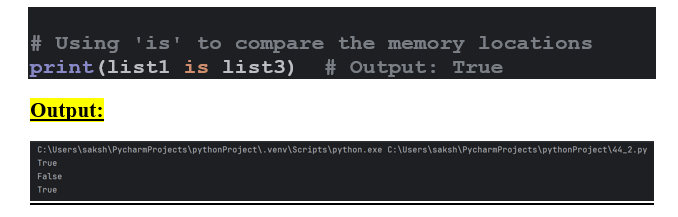
Key Differences:
- == compares the values of two variables.
- is compares the memory locations of two variables.
Best Practices:
- Use == when comparing the values of variables.
- Use is when checking for identity, i.e., when you want to check if two variables refer to the same object in memory.
Understanding the distinction between is and == is important for writing correct and efficient Python code, especially when dealing with mutable objects like lists, dictionaries, and custom objects.
@SAKSHAM DIXIT