Lazy Programming Series – Classes and Objects In Python
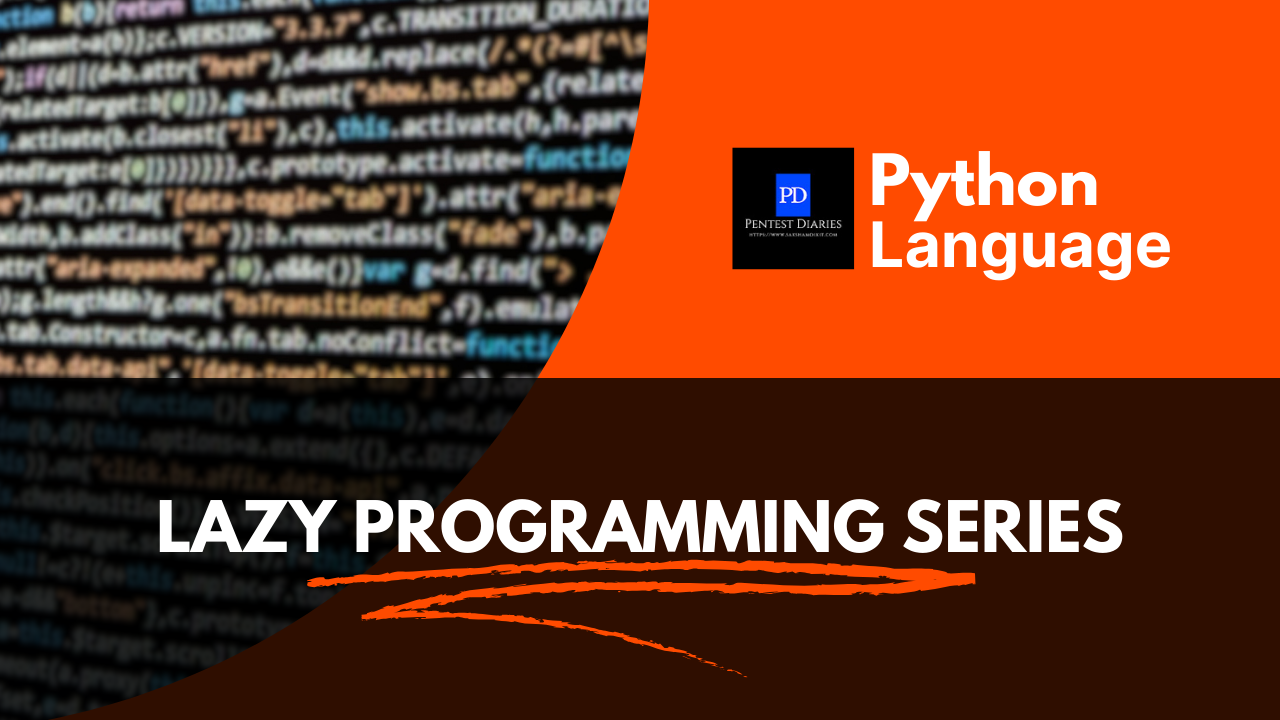
Classes and Objects In Python:
Classes and objects are fundamental concepts in object-oriented programming (OOP), and Python supports OOP paradigms very well. Here’s an overview of what classes and objects are, and how to define and use them in Python.
Classes
A class is a blueprint for creating objects. It defines a set of attributes and methods that the created objects (instances of the class) will have.
Defining a Class
In Python, you define a class using the class keyword. Here’s a basic example:
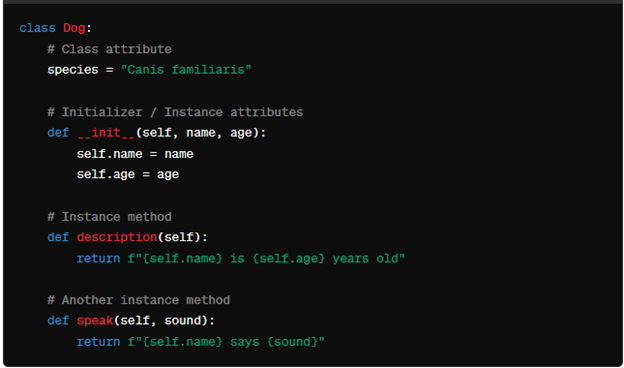
Objects
An object is an instance of a class. When a class is defined, no memory is allocated until an object of that class is created. Objects are the real-world entities created using the class blueprint.
Creating an Object
You create an object by calling the class as if it were a function:

This line of code creates an instance of the Dog
class named my_dog
with the name “Buddy” and age 3.
Accessing Attributes and Methods
Once you have an object, you can access its attributes and methods using dot notation:
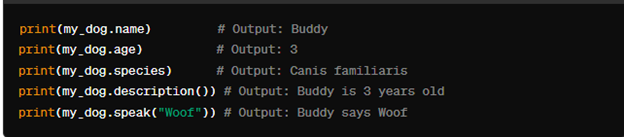
Example: Full Code
Here’s a complete example of putting it all together:
CODE:
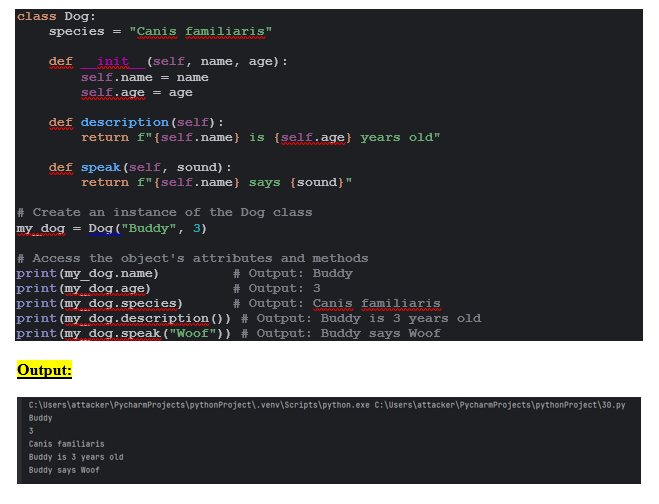
Key Concepts
- Class Attributes: Variables that are shared among all instances of a class. Defined within the class but outside any instance methods.
- Instance Attributes: Variables that are unique to each instance. Defined within the
__init__
method. - Methods: Functions defined within a class that describe the behaviors of the objects. They take at least one parameter,
self
, which refers to the instance calling the method.
Special Methods
Python classes have special methods, often referred to as magic methods, that start and end with double underscores. These methods can override default behaviors. For example:
__init__
: Initializes a new instance of the class.__str__
: Returns a string representation of the object, used by theprint
function.__repr__
: Returns an official string representation of the object.
Example with __str__
and __repr__
:
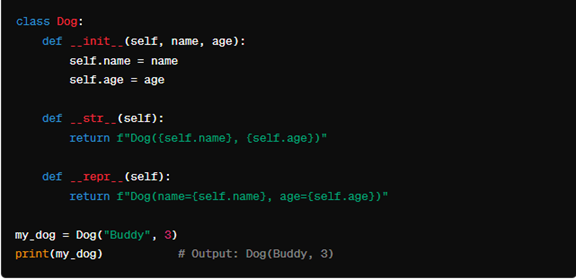
Understanding and using classes and objects allows for better structuring of your code and facilitates code reuse, scalability, and maintainability.
@SAKSHAM DIXIT