Lazy Programming Series – Global , Local & nonlocal Scope
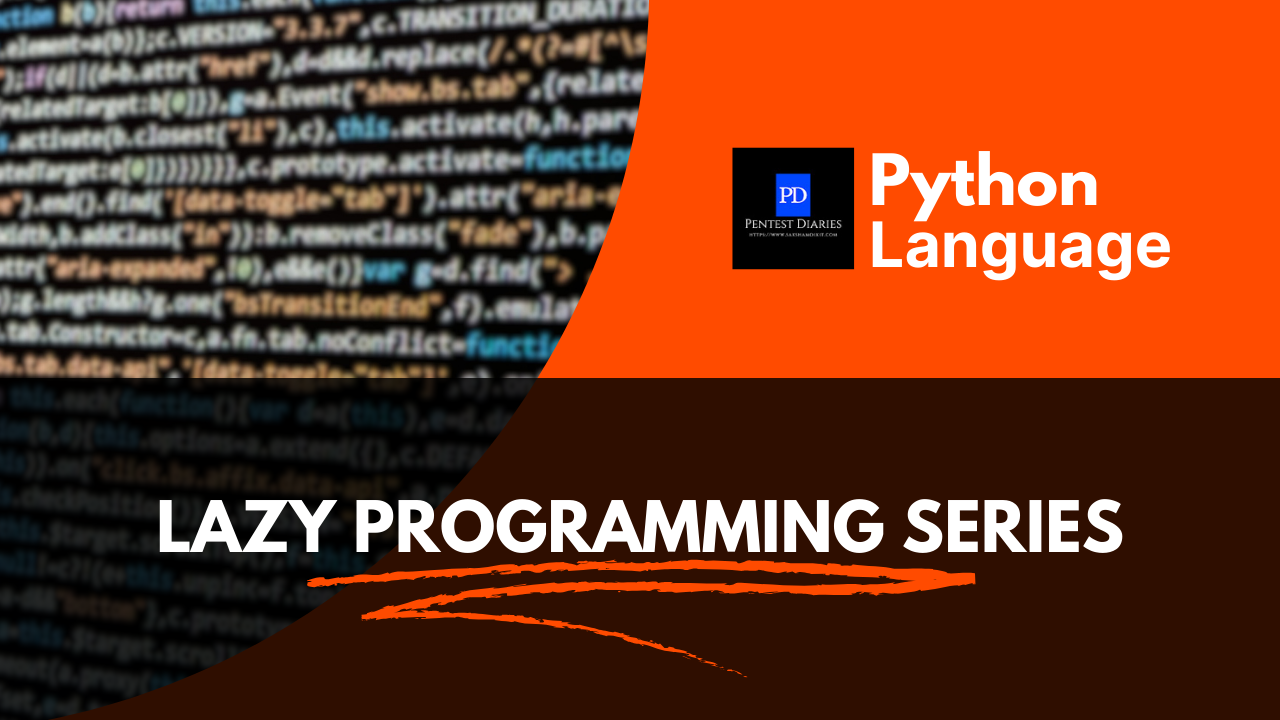
In Python, the term “scope” refers to the region of a program where a particular variable is accessible. Python has four main scopes:
- Local scope: Variables defined within a function have local scope. They can only be accessed within that function.
- Enclosing scope: If a function is defined within another function, the inner function has access to variables in the outer (enclosing) function’s scope.
- Global scope: Variables defined at the top level of a module or explicitly declared as global have global scope. They can be accessed from anywhere within the module.
- Built-in scope: Python has a set of built-in functions and types that are always available.
Global variables in Python are variables that are declared outside of any function or in global scope. They can be accessed and modified from any part of the program.
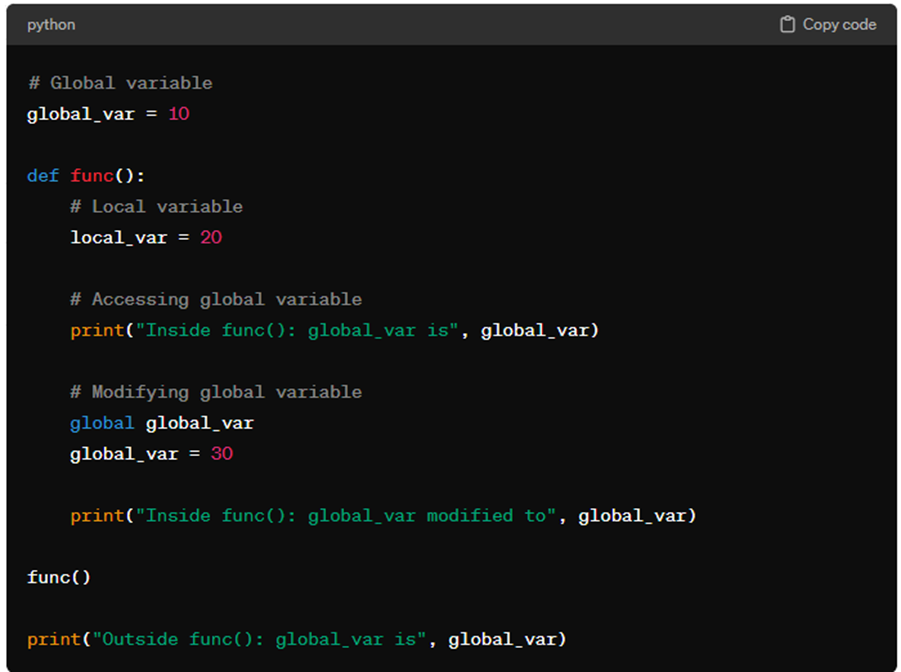
In this example:
- global_var is a global variable accessible from within the function func().
- local_var is a local variable only accessible within func().
- We use the global keyword inside func() to modify the value of global_var.
Output:
In this example:
- global_var is a global variable accessible from within the function func().
- local_var is a local variable only accessible within func().
- We use the global keyword inside func() to modify the value of global_var.
Output:
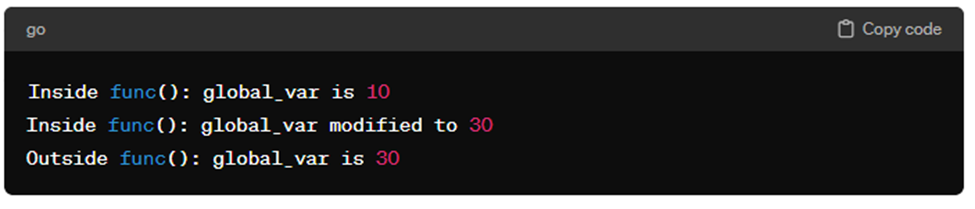
Using the global
keyword allows us to modify global variables from within a function. However, it’s generally considered a good practice to avoid global variables when possible, as they can make code harder to understand and maintain.
A global variable cannot be changed in function as a global variable.
If we want to change global then define that variable in function as global.
Code:
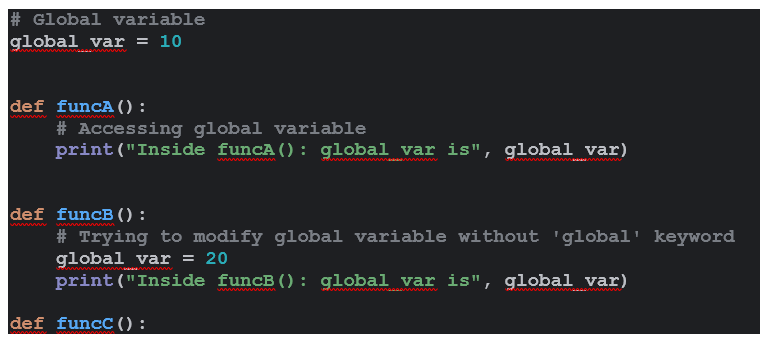
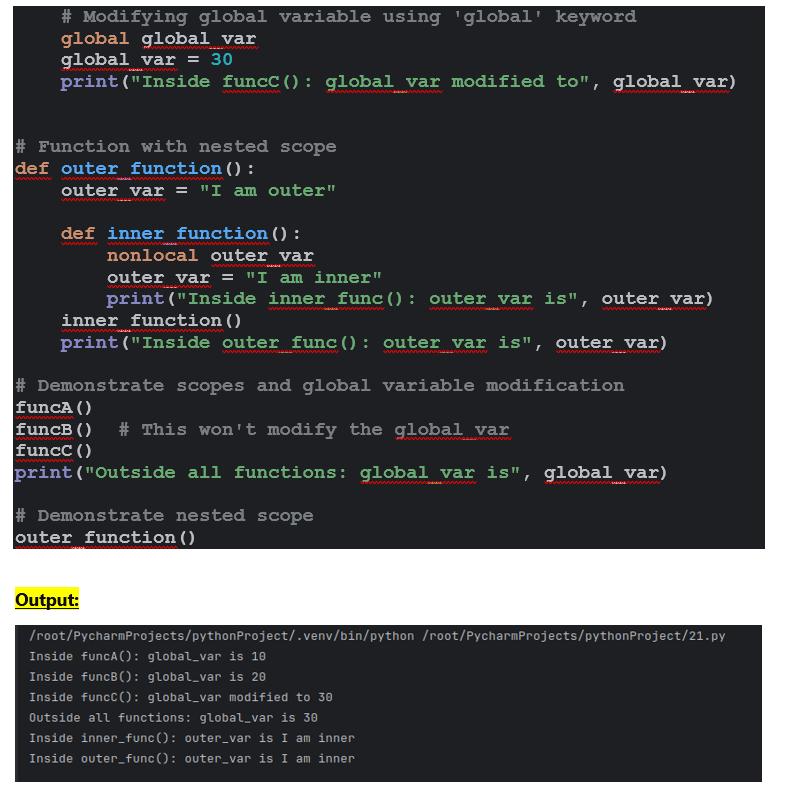