Lazy Programming Series – Operators in Python
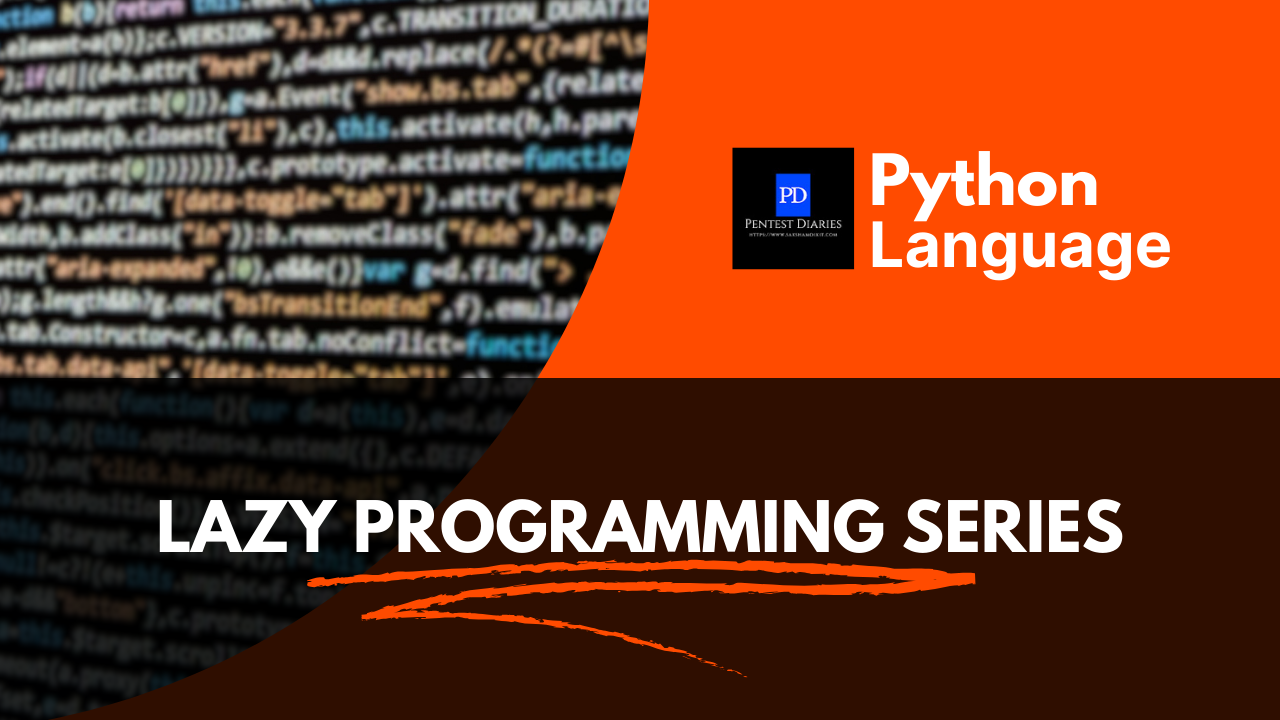
In Python, operators are special symbols or keywords that are used to perform operations on variables and values. Here’s a list of some common operators in Python:
- Arithmetic Operators:
- Addition (+)
- Subtraction (-)
- Multiplication (*)
- Division (/)
- Floor Division (//) – Returns the integer part of the quotient
- Modulus (%) – Returns the remainder of the division
- Exponentiation (**) – Raises a number to a power
- Comparison (Relational) Operators:
- Equal to (==)
- Not equal to (!=)
- Greater than (>)
- Less than (<)
- Greater than or equal to (>=)
- Less than or equal to (<=)
- Logical Operators:
- Logical AND (and)
- Logical OR (or)
- Logical NOT (not)
- Assignment Operators:
- Assign (=)
- Add and assign (+=)
- Subtract and assign (-=)
- Multiply and assign (*=)
- Divide and assign (/=)
- Modulus and assign (%=)
- Exponentiate and assign (**=)
- Floor divide and assign (//=)
- Identity Operators:
- is – Returns true if both variables are the same object
- is not – Returns true if both variables are not the same object
- Membership Operators:
- in – Returns true if a sequence with the specified value is present in the object
- not in – Returns true if a sequence with the specified value is not present in the object
- Bitwise Operators:
- Bitwise AND (&)
- Bitwise OR (|)
- Bitwise XOR (^)
- Bitwise NOT (~)
- Left Shift (<<)
- Right Shift (>>)
These operators can be used in combination with variables, values, and expressions to perform various operations like arithmetic calculations, comparisons, logical operations, etc.
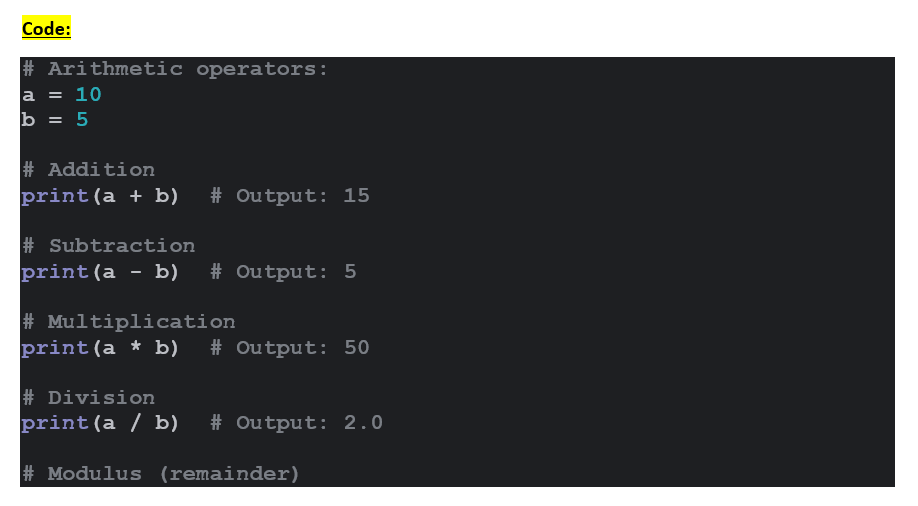
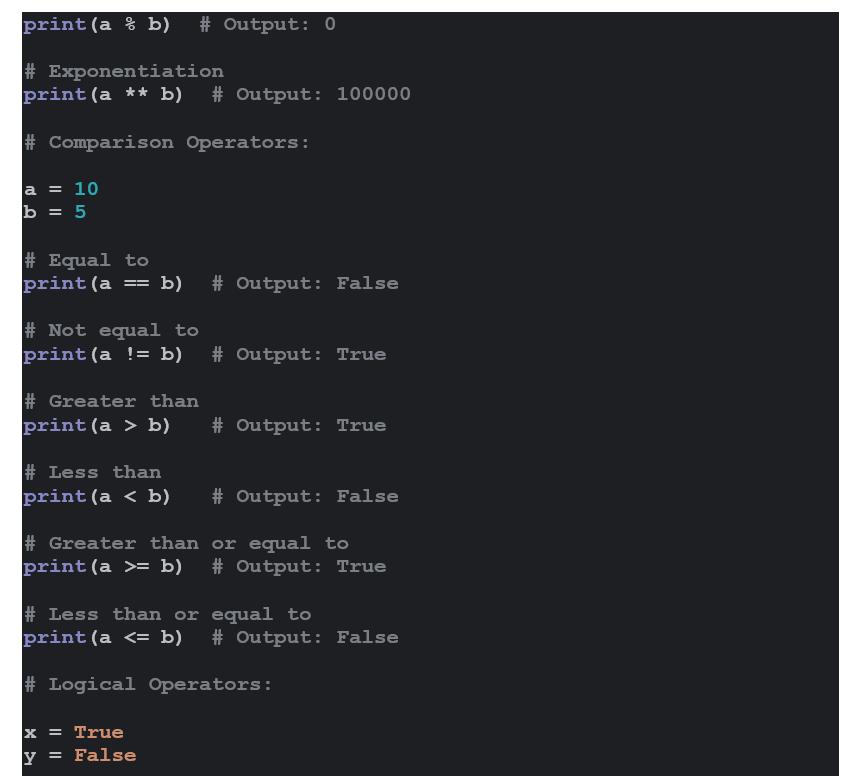
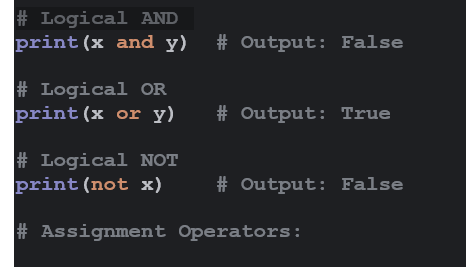
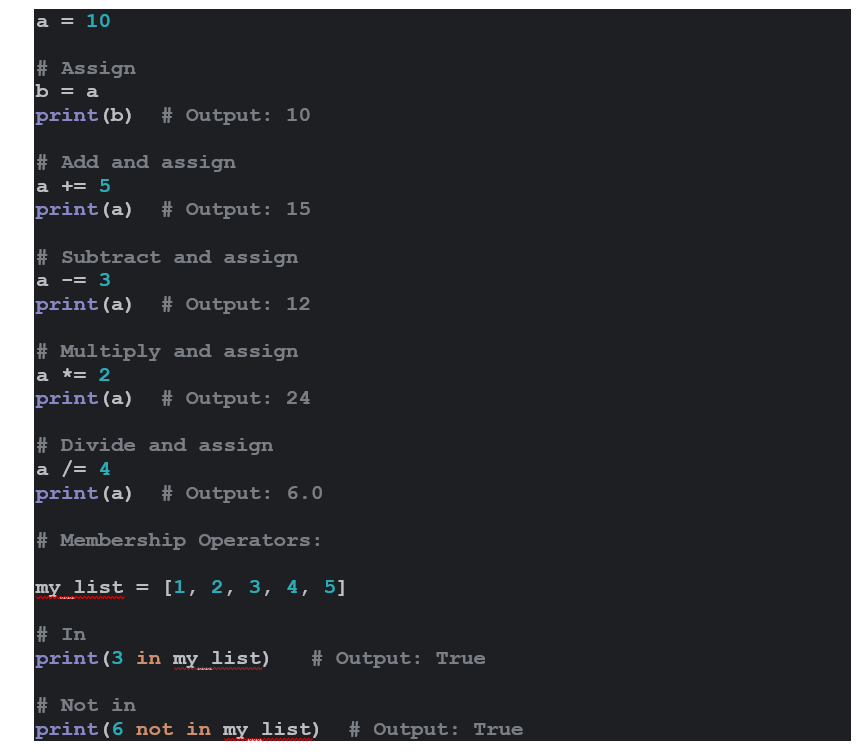
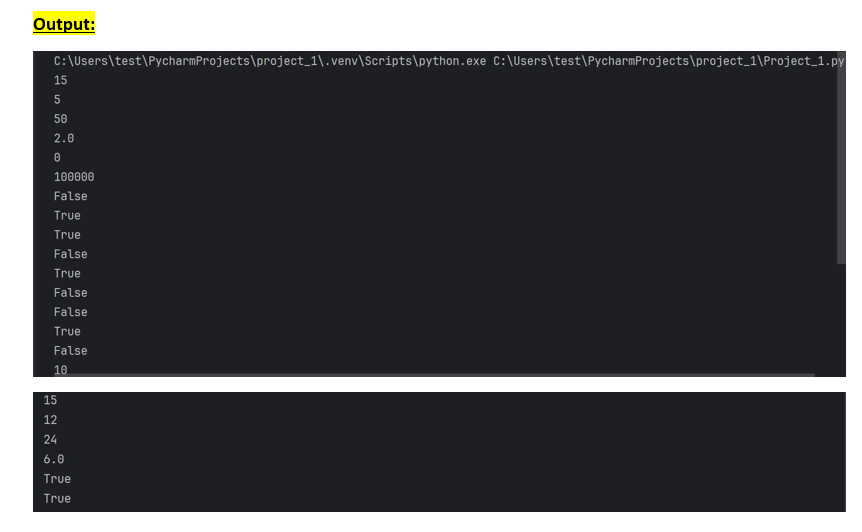