Lazy Programming Series – Self , __init__() constructor , Class method , CM alternative constructors
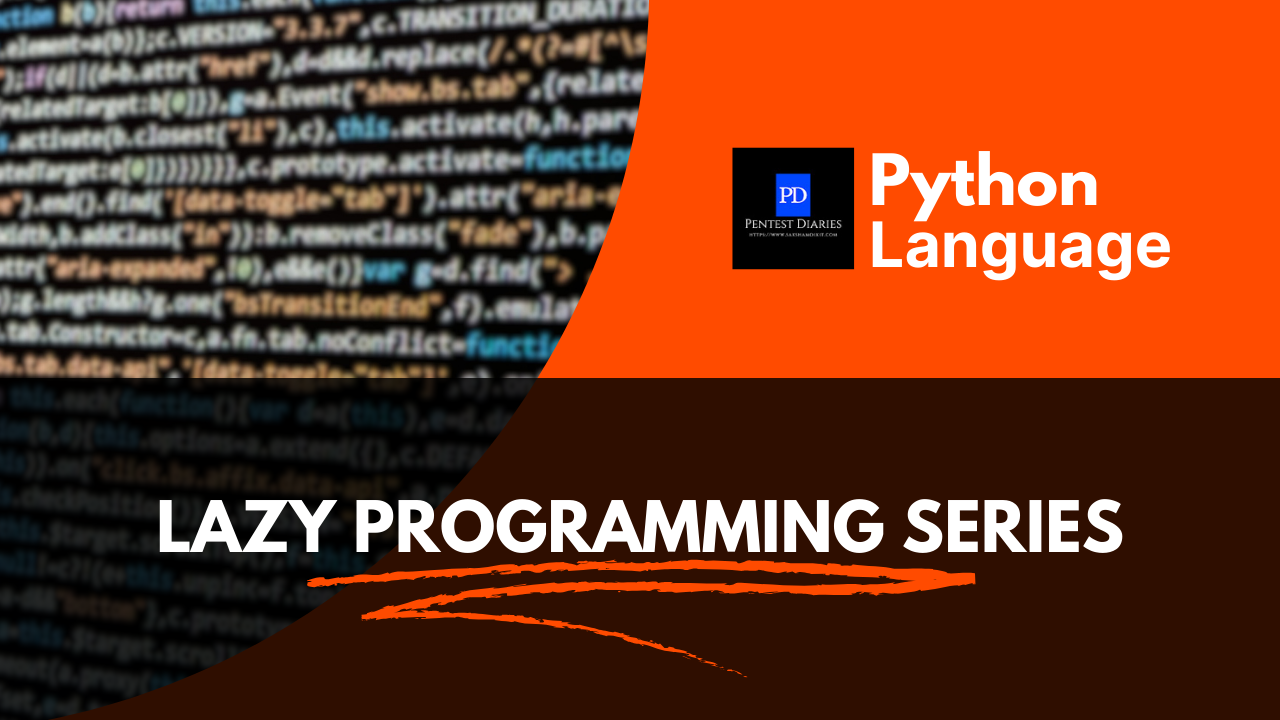
Self & __init__() (constructor) in Python:
In Python, self
and __init__()
are fundamental concepts used in defining and initializing class instances.
self
self
is a reference to the current instance of the class. It is used to access variables and methods associated with the instance. In a class’s method, self
allows you to refer to instance variables and methods from within that method.
Usage of self
- Instance Variables:
self
is used to define and access instance variables. - Methods:
self
is used to call other methods within the same class.
Example:
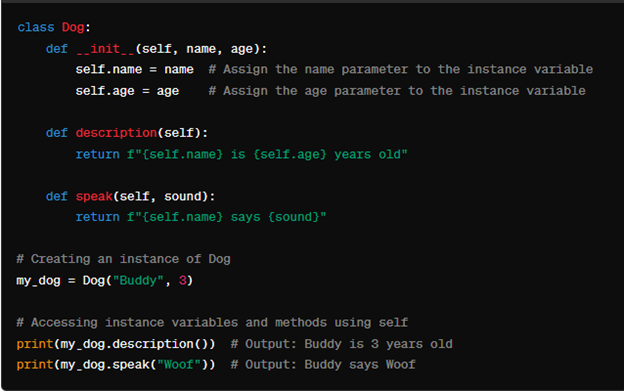
In this example, self
is used to assign the parameters name
and age
to the instance variables self.name
and self.age
, and to refer to them within the methods description
and speak
.
__init__()
(Constructor)
__init__()
is a special method in Python classes, known as the constructor. It is called when an instance (object) of the class is created. The __init__()
method initializes the instance by setting up initial values for instance variables or performing any other necessary setup.
Defining __init__()
The __init__()
method is defined with the def
keyword, just like any other method. The first parameter is always self
, followed by any additional parameters that you want to use for initializing the instance.
Example:
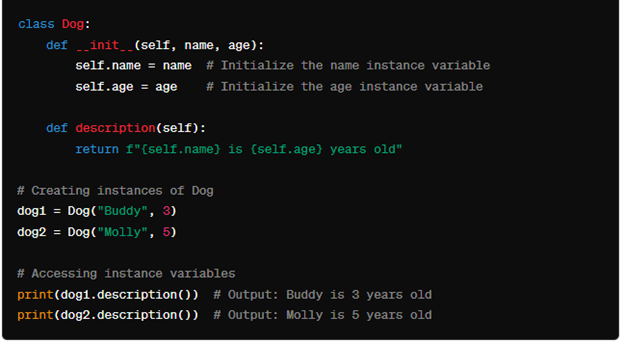
In this example, __init__() initializes the name and age instance variables for each Dog instance.
Key Points
- self:
- Refers to the instance calling the method.
- Allows access to instance variables and other methods.
- Always the first parameter in instance methods.
- __init__():
- Special method for initializing new instances.
- Called automatically when a new instance is created.
- Takes self and other parameters to set up the instance.
Example: Complete Class with self and __init__()
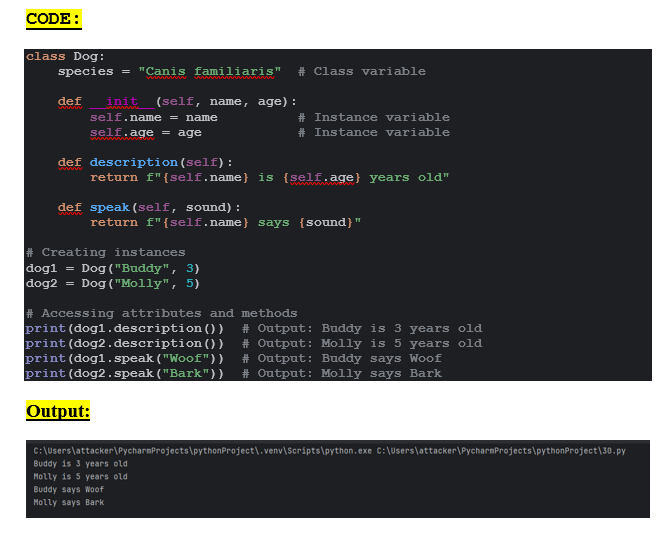
Class methods in Python:
In Python, class methods are methods that are bound to the class itself rather than to an instance of the class. They can access and modify the class state that applies across all instances of the class. Class methods are defined using the @classmethod decorator, and they take cls as the first parameter to refer to the class (similar to how instance methods take self as the first parameter to refer to the instance).
Defining and Using Class Methods
To define a class method, you use the @classmethod decorator and include cls as the first parameter in the method definition. Here’s an example to illustrate:
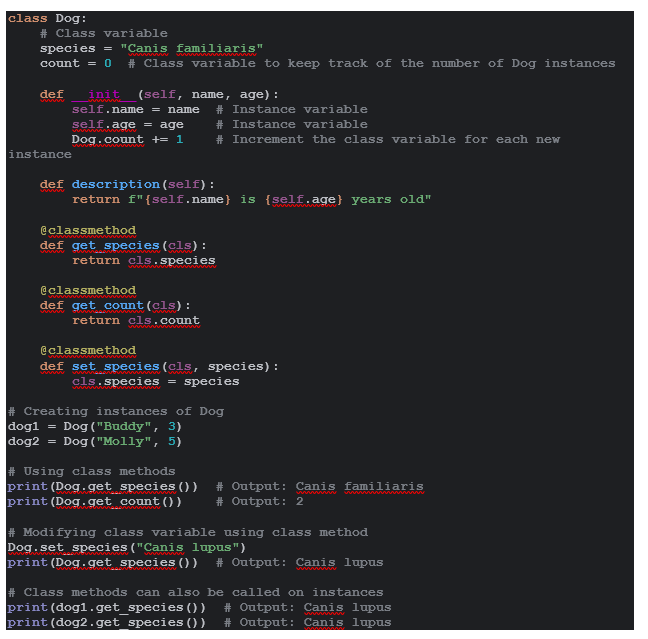
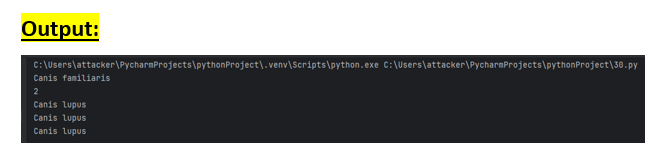
Key Points about Class Methods
- Class Methods vs. Instance Methods:
- Class Methods: Use
cls
to access class-level data and methods. They are called on the class itself or on instances. - Instance Methods: Use
self
to access instance-level data and methods. They are called on instances of the class. - Class Method Use Cases:
- Accessing or modifying class variables.
- Creating factory methods that return an instance of the class, possibly with some pre-defined settings.
Example of a Factory Method
Factory methods are class methods that return an instance of the class. They can be used to create instances in a more controlled manner.
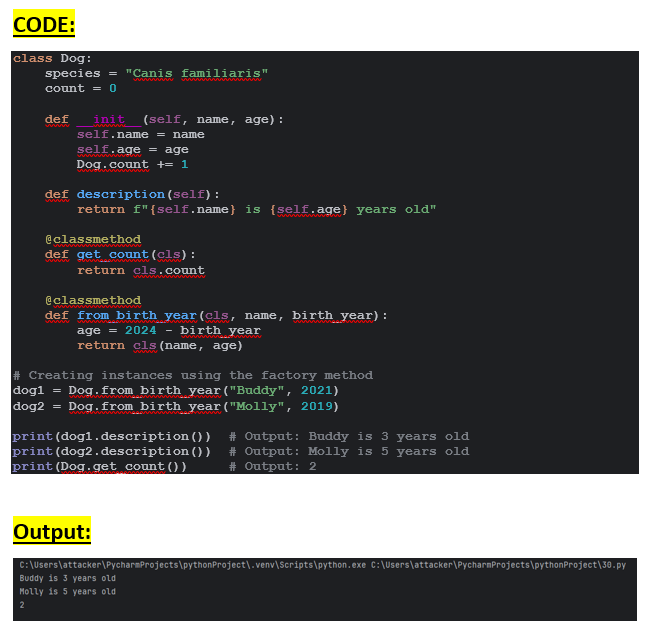
Class Methods as alternative constructors in python
Class methods can be used as alternative constructors in Python. These alternative constructors are class methods that provide different ways to create instances of the class. This can be particularly useful for initializing objects from various data sources or formats.
Using Class Methods as Alternative Constructors
To create an alternative constructor, you define a class method with the @classmethod decorator. These methods typically perform some kind of preprocessing or setup before calling the primary constructor (__init__) of the class.
Example: Alternative Constructors
Here is an example using a Dog class with alternative constructors:
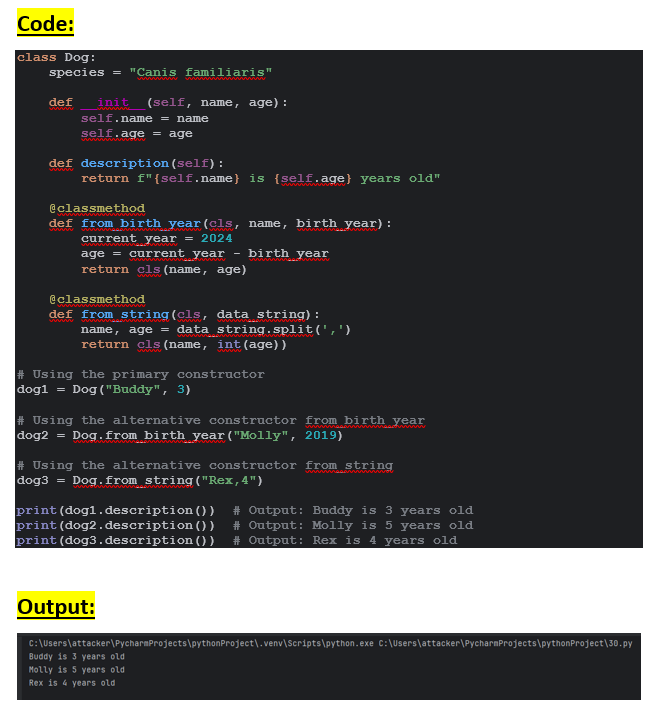
Benefits of Using Alternative Constructors
- Encapsulation: Alternative constructors can encapsulate complex initialization logic and keep it out of the main constructor.
- Flexibility: They provide different ways to create instances, making your class more flexible and easier to use with various data sources.
- Clarity: Named constructors like
from_birth_year
andfrom_string
make the code more readable and expressive, indicating exactly how the instance is being created.
Another Example: A Class with Multiple Alternative Constructors
Consider a Book
class with alternative constructors for creating instances from different formats:
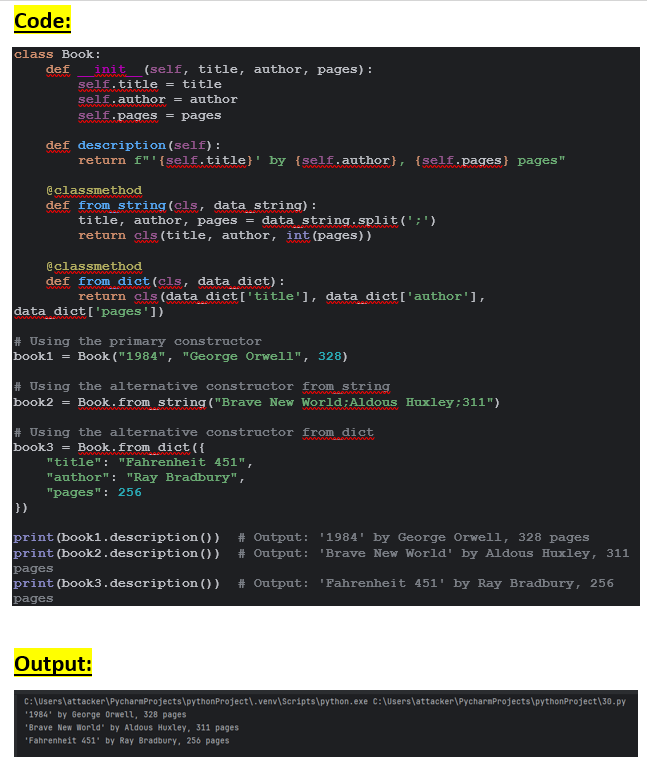
@SAKSHAM DIXIT