Lazy Programming Series – Static Method, Abstraction & Encapsulation in Python
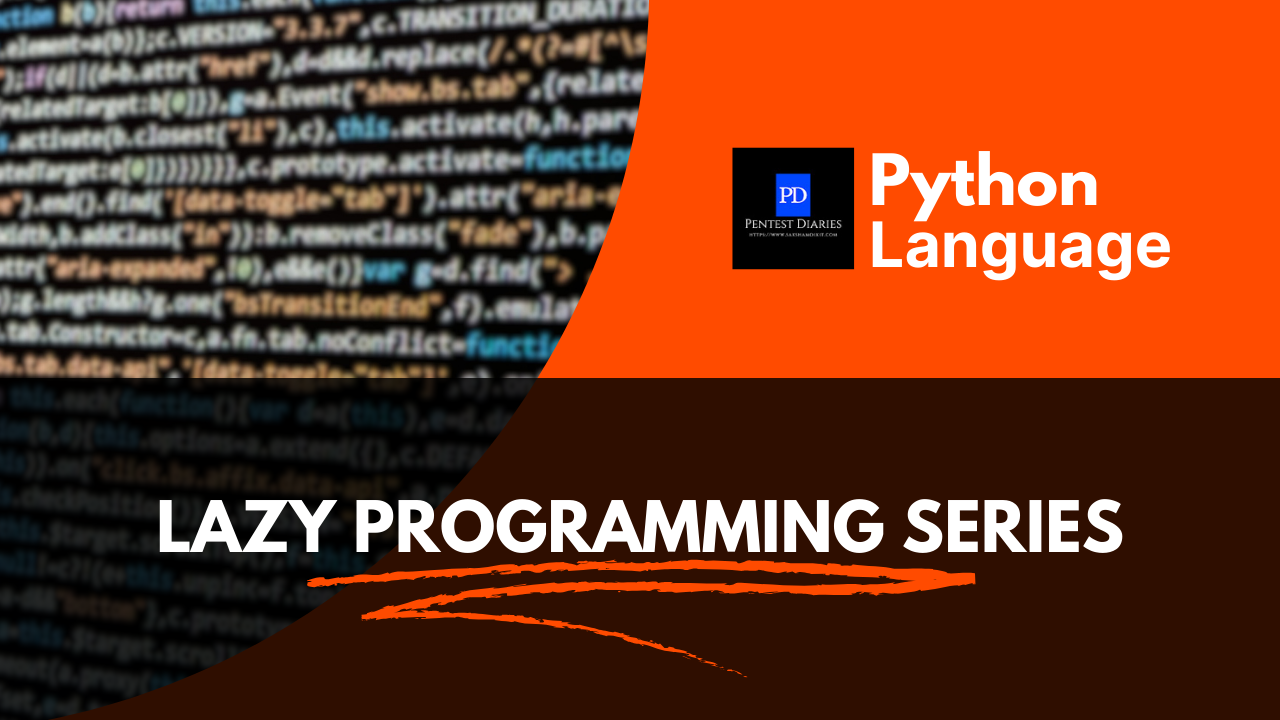
Static Methods in Python:
In Python, static methods are methods that are bound to a class rather than an instance of the class. They don’t require access to the instance or class attributes and can be called without creating an instance of the class. You define a static method using the @staticmethod
decorator.
Here’s an example:
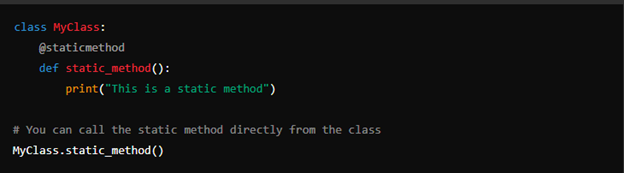
Static methods are often used for utility functions that don’t depend on instance or class state but are related to the class conceptually.
For example, in a MathUtils
class, you might have a static method to compute the square of a number:
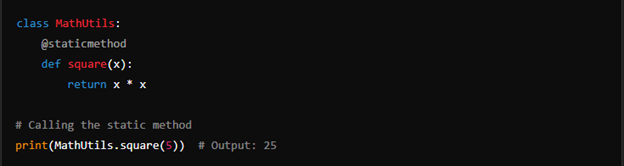
Remember, static methods don’t have access to instance or class variables unless explicitly passed as arguments. They’re essentially just like regular functions but scoped within the class namespace.
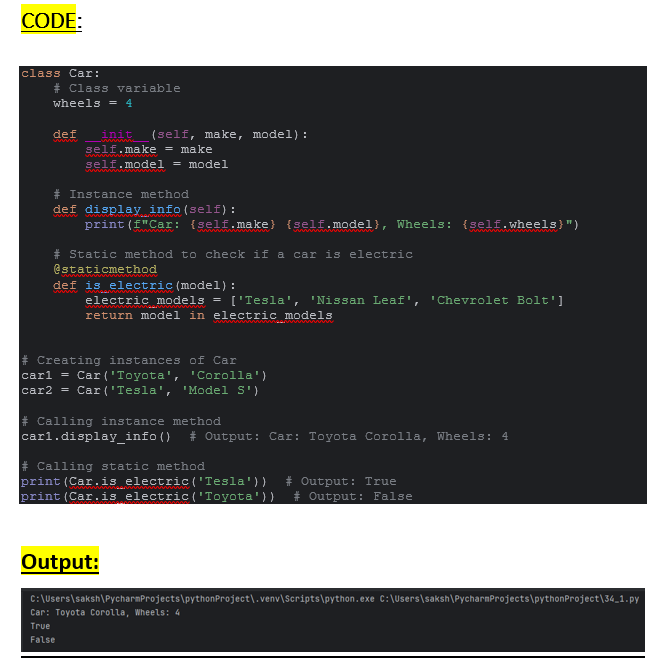
Abstraction & Encapsulation:
Abstraction and encapsulation are two important concepts in object-oriented programming, including Python.
Abstraction: Abstraction is the process of hiding the complex implementation details and showing only the necessary features of an object to the outside world. It allows you to focus on what an object does rather than how it does it. In Python, abstraction can be achieved through abstract classes and interfaces, as well as through method signatures.
Here’s a simple example demonstrating abstraction using Python’s abstract base classes (ABCs) from the abc
module:
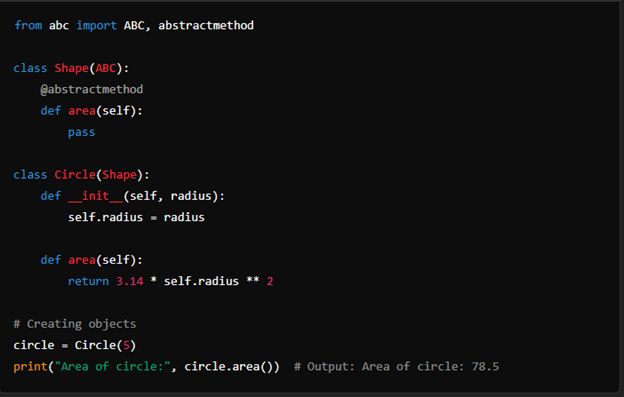
In this example, the Shape
class defines an abstract method area()
, which any concrete subclass must implement. The Circle
class is a concrete implementation of Shape
, providing its own implementation of the area()
method.
Encapsulation: Encapsulation is the bundling of data (attributes) and methods that operate on that data into a single unit, often referred to as a class. It hides the internal state of an object from the outside world and only exposes the necessary functionalities through methods. Encapsulation helps in achieving data abstraction and prevents direct access to the data, thereby ensuring data integrity and security.
Here’s a simple example demonstrating encapsulation in Python:
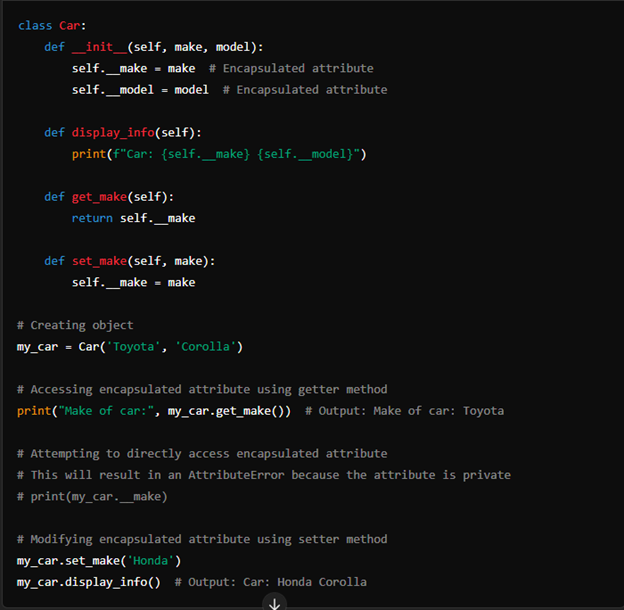
In this example, __make
and __model
are encapsulated attributes of the Car
class, which are accessed and modified through getter and setter methods (get_make()
and set_make()
). This encapsulation hides the internal state of the Car
object and provides controlled access to its attributes.
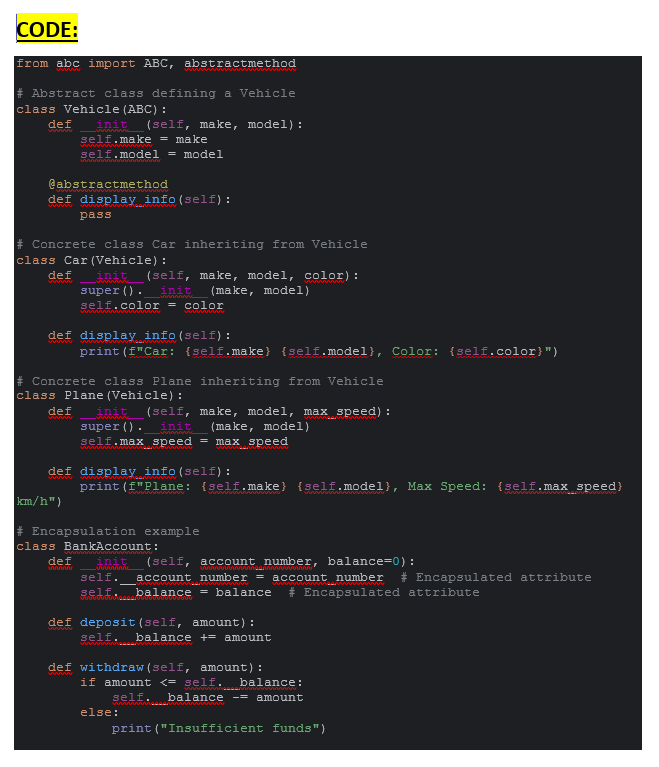
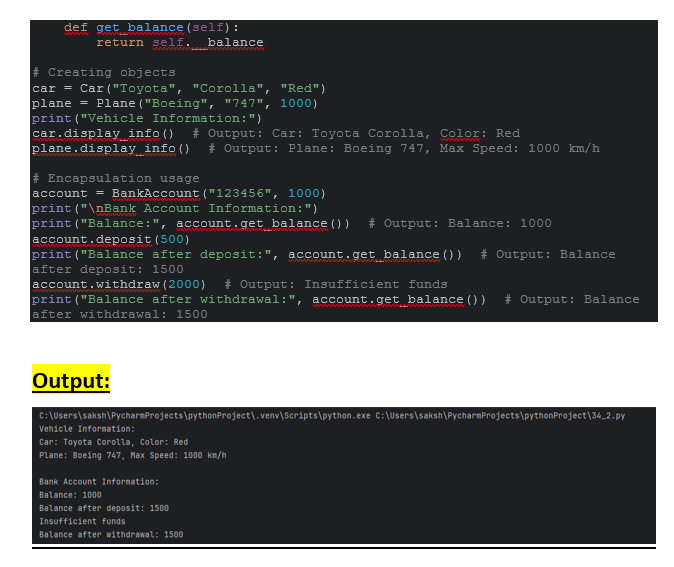
@SAKSHAM DIXIT