Lazy Programming Series – Super(), Multiple Inheritance, Dunder Method, Abstract base class & @abstractmethod, Setters & Property Decorators, Object Introspection In Python
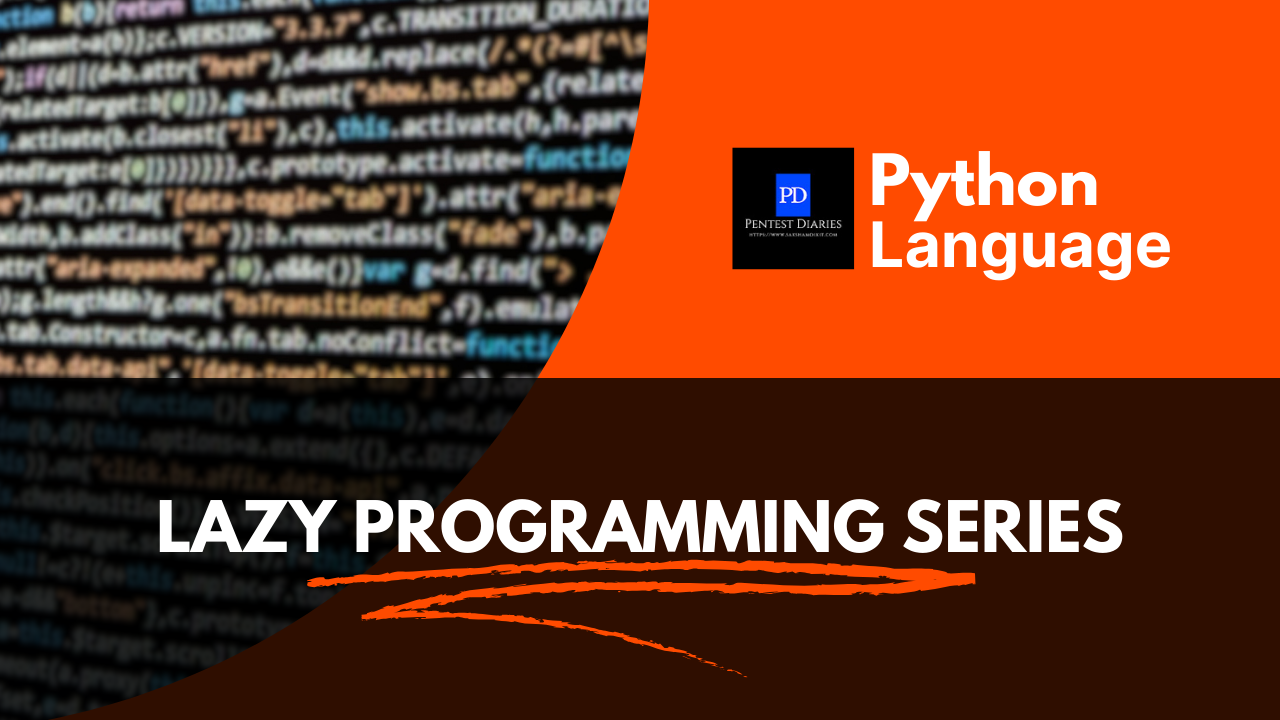
SUPER() in Classes:
Certainly! super()
in Python is used to access methods and properties from a parent class. It’s particularly useful in situations where you’re extending a class (subclassing) and want to invoke the methods or properties of the parent class.
Here’s how super()
works:
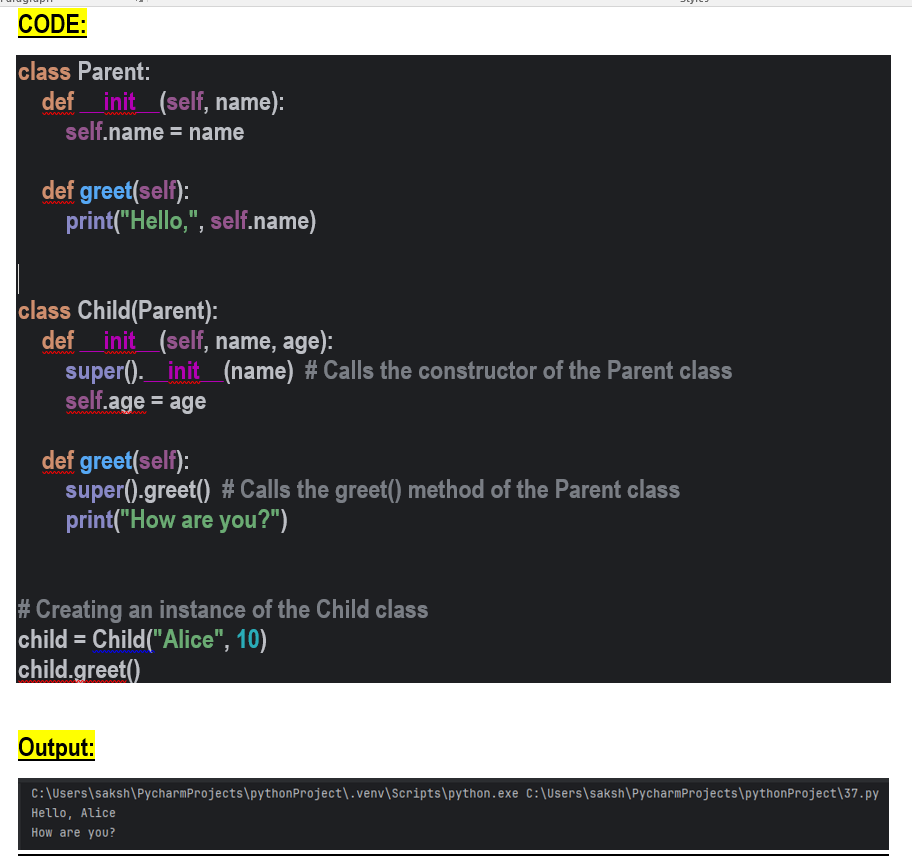
DIAMOND SHAPE PROBLEM IN MULTIPLE INHERITANCE IN PYTHON:
The Diamond Shape Problem is a common issue that arises in programming languages that support multiple inheritance, including Python. It occurs when a class inherits from two or more classes that have a common ancestor. As a result, there can be ambiguity in method resolution or attribute lookup, leading to unexpected behavior.
Here’s a simplified example to illustrate the Diamond Shape Problem:
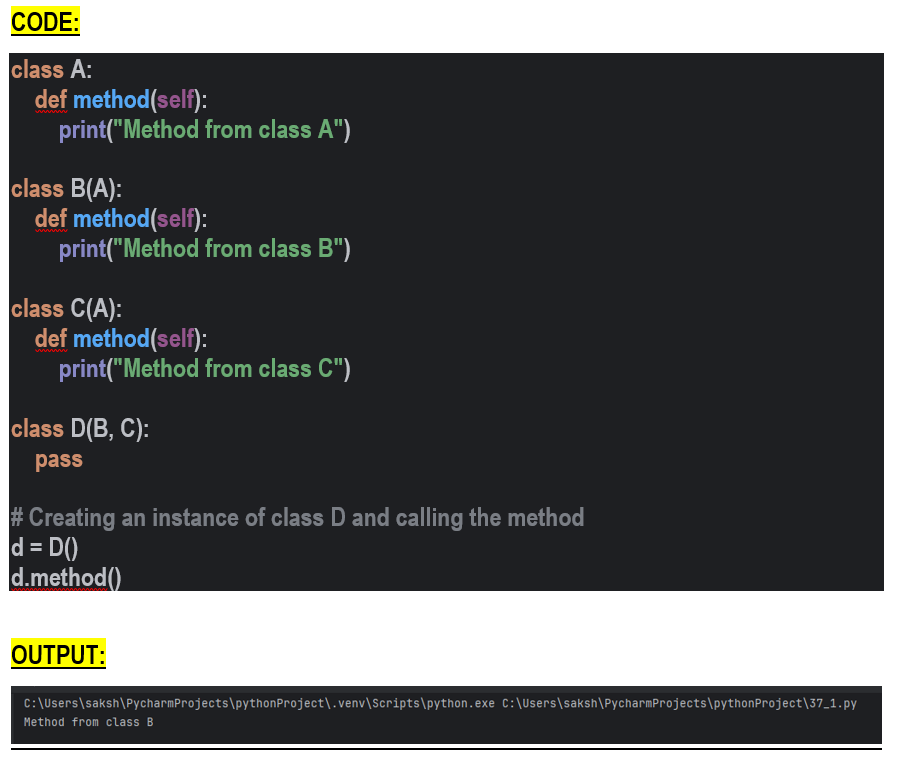
DUNDER METHODS IN PYTHON:
Dunder methods, short for “double underscore” methods, are special methods in Python that have names surrounded by double underscores on both sides. These methods are also known as magic methods or special methods. Dunder methods allow classes to define how they interact with Python’s built-in functionality, such as arithmetic operations, comparison operators, and built-in functions like len()
, str()
, repr()
, etc.
Here are a few commonly used dunder methods:
__init__(self, ...)
: The constructor method, called when an object is instantiated.__str__(self)
: Called by thestr()
function andprint()
to return a string representation of an object.__repr__(self)
: Called by therepr()
function to return a string representation of an object for debugging purposes.__len__(self)
: Called by thelen()
function to return the length of an object.__getitem__(self, key)
: Called to retrieve an item from an object using square bracket notation (obj[key]
).__setitem__(self, key, value)
: Called to set an item in an object using square bracket notation (obj[key] = value
).__delitem__(self, key)
: Called to delete an item from an object using thedel
statement (del obj[key]
).__iter__(self)
: Called when an object is iterated over, for example, in afor
loop.__next__(self)
: Called to return the next item in an iterator.__eq__(self, other)
: Called when testing for equality using the==
operator.__lt__(self, other)
,__le__(self, other)
,__gt__(self, other)
,__ge__(self, other)
: Comparison methods for less than, less than or equal to, greater than, and greater than or equal to, respectively.
These dunder methods allow Python classes to define custom behavior for various operations and integrate seamlessly with Python’s syntax and built-in functions. By implementing these methods, you can make your custom objects behave like built-in types, providing a more intuitive and consistent interface for users of your code.
ABSTRACT BASE CLASS & @abstractmethod:
Abstract Base Classes (ABCs) in Python are a way of defining abstract interfaces for classes. They provide a blueprint for other classes to follow by specifying a set of methods that must be implemented by any concrete subclass. ABCs help enforce a common interface across different implementations, making code more predictable and maintainable.
In Python, the abc module provides support for defining abstract base classes. The @abstractmethod decorator is used to mark methods within an ABC that must be implemented by concrete subclasses. If a subclass fails to implement one or more abstract methods defined in the ABC, Python raises a TypeError at runtime.
Here’s an example illustrating the usage of ABCs and @abstractmethod:
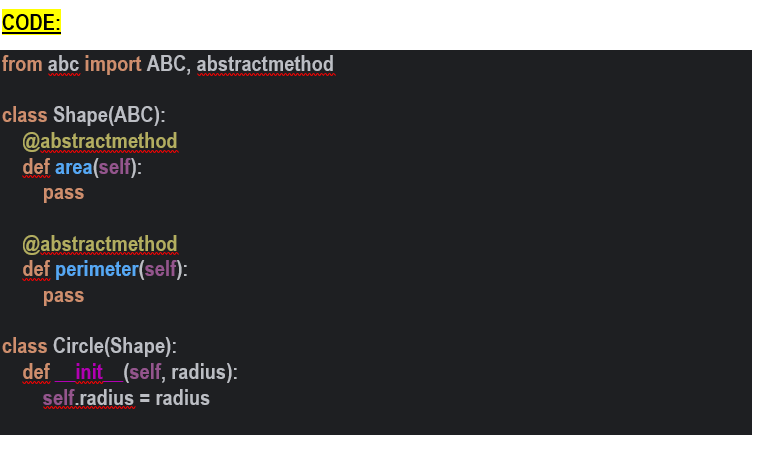
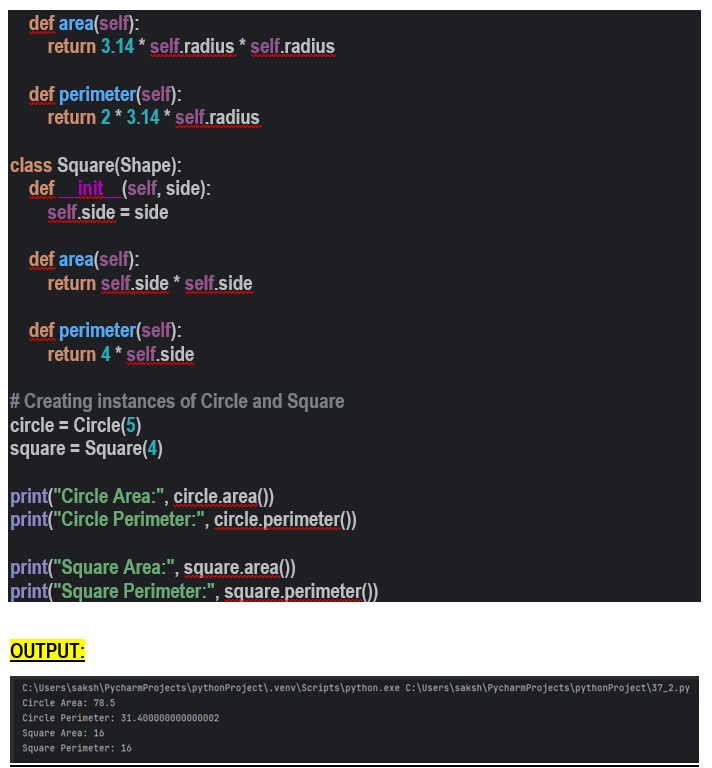
In this example:
Shape is an abstract base class that defines two abstract methods: area() and perimeter().
Circle and Square are concrete subclasses of Shape. They implement the area() and perimeter() methods, as required by the ABC.
If you attempt to instantiate a subclass without implementing all the abstract methods, Python will raise a TypeError.
Abstract base classes and @abstractmethod provide a powerful mechanism for defining interfaces and enforcing adherence to those interfaces in Python code. They promote code reusability, maintainability, and help catch errors at compile time rather than runtime.
SETTERS & PROPERTY DECORATORS:
In Python, setters and property decorators are used to implement property accessors and mutators, allowing you to define custom behavior for getting and setting attributes of an object.
Property Decorator: The property
decorator allows you to define a method as a property of a class. It provides a way to customize attribute access, allowing you to execute code when accessing or assigning values to an attribute.
Here’s how you can use the property
decorator:
In this example, x
is a property of the MyClass
class. The @property
decorator marks the x()
method as a getter, and @x.setter
decorator marks the x()
method as a setter. When you access obj.x
, it calls the getter method, and when you assign a value to obj.x
, it calls the setter method.
Setters: Setters are methods that are used to set the value of an attribute in a class. They allow you to perform validation or execute additional code when setting the value of an attribute.
Here’s how you can define a setter without using the property decorator:
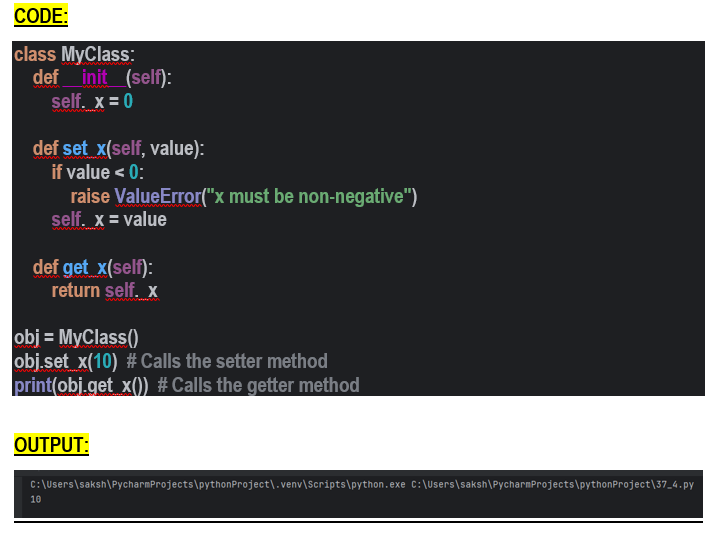
In this example, set_x() is a setter method that sets the value of _x, and get_x() is a getter method that returns the value of _x.
Using property decorators and setters allows you to define properties with custom behavior, such as validation or computed attributes, making your classes more flexible and easier to work with.
Top of Form
OBJECT INTROSPECTION:
Object introspection in Python refers to the ability to examine the attributes and methods of an object at runtime. It allows you to programmatically inspect the structure, properties, and behavior of objects in your code. Python provides several built-in functions and techniques for object introspection:dir()
Function: The dir()
function returns a list of attributes and methods of an object. It provides a comprehensive view of the object’s structure.
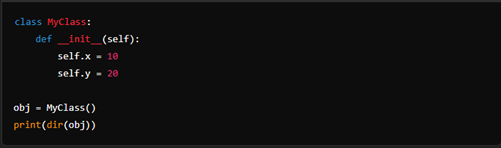
type()
Function: The type()
function returns the type of an object. It’s useful for determining the class of an object.

isinstance()
Function: The isinstance()
function checks if an object is an instance of a particular class or type.

getattr()
Function: The getattr()
function retrieves the value of an attribute of an object by name.
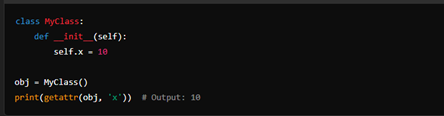
hasattr()
Function: The hasattr()
function checks if an object has a particular attribute.
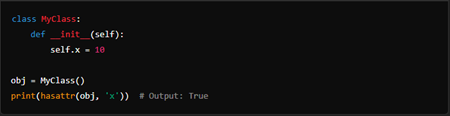
setattr()
Function: The setattr()
function sets the value of an attribute of an object by name.
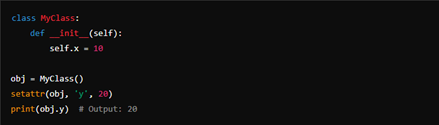

@SAKSHAM DIXIT