Lazy programming Series – Variables, Data Types & Typecasting | Practical Code
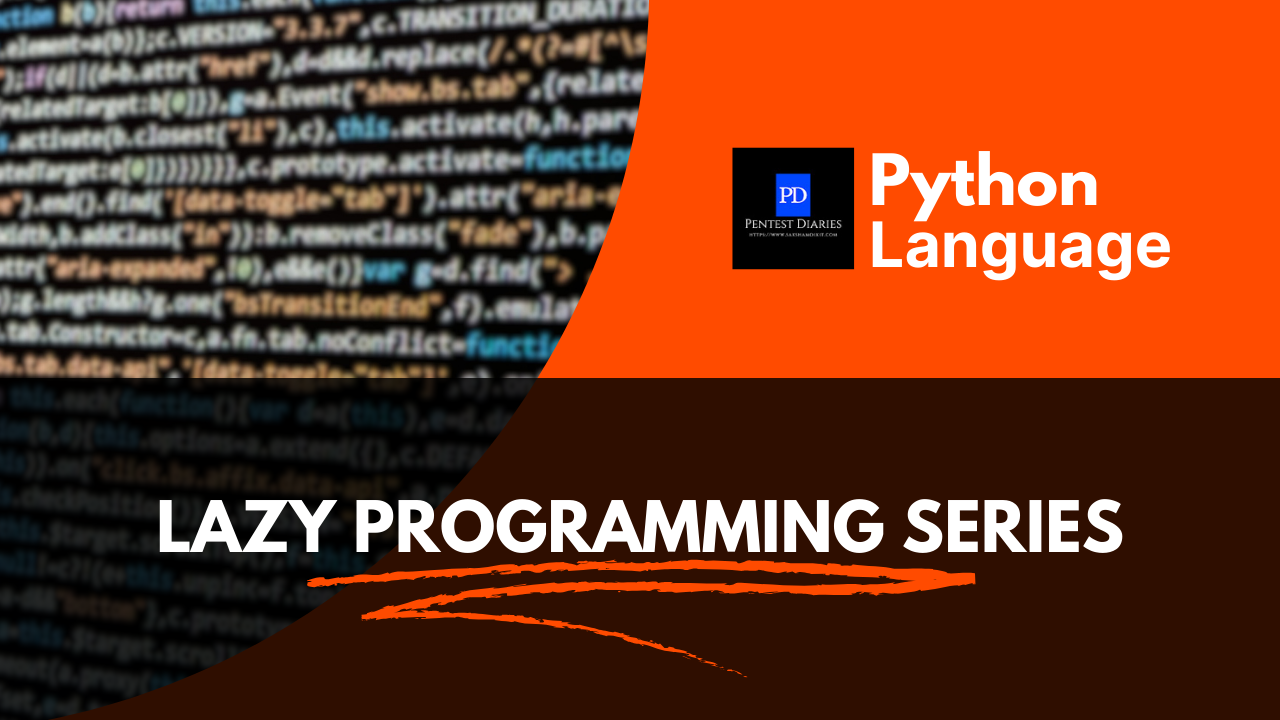
In Python, variables are used to store data values. Each variable has a data type, which defines the type of data it can hold. Here’s a brief overview of variables, data types, and typecasting in Python:
Variables:
Variables are containers for storing data values. You can think of them as named storage locations that can hold different types of data. In Python, you don’t need to explicitly declare the data type of a variable; the interpreter infers it based on the assigned value.
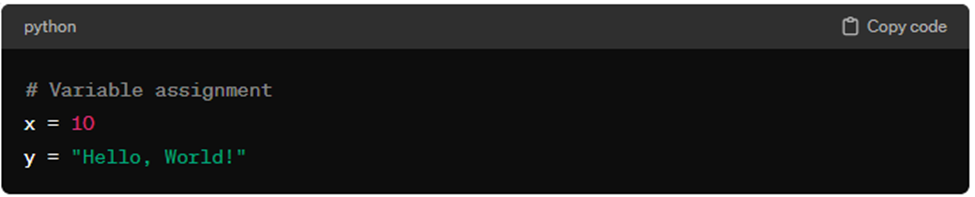
Data Types:
Python supports various built-in data types. Some common ones include:
Numeric Types:
int
: Integer type (e.g., 5, -10)float
: Floating-point type (e.g., 3.14, -2.5)
Text Type:
str
: String type (e.g., “Hello”, ‘Python’)
Boolean Type:
bool
: Boolean type (e.g., True, False)
Sequence Types:
list
: List type (e.g., [1, 2, 3])tuple
: Tuple type (e.g., (1, 2, 3))
range
: Range type (e.g., range(0, 5))
Mapping Type:
dict
: Dictionary type (e.g., {‘key’: ‘value’})
Set Types:
set
: Set type (e.g., {1, 2, 3})
Typecasting:
Typecasting involves converting a variable from one data type to another. Python provides several built-in functions for typecasting:
int()
, float()
, str()
:Convert to integer, float, or string, respectively.

list(), tuple(), set():
- Convert to list, tuple, or set, respectively.

bool():
- Convert to boolean.

Remember to use typecasting carefully, as it may result in loss of data or unexpected behavior if not done correctly. It’s always a good practice to check the type of a variable before performing typecasting.
Code:
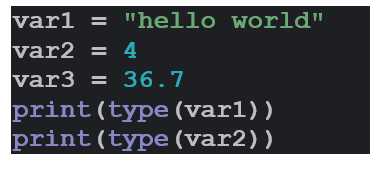

Output:
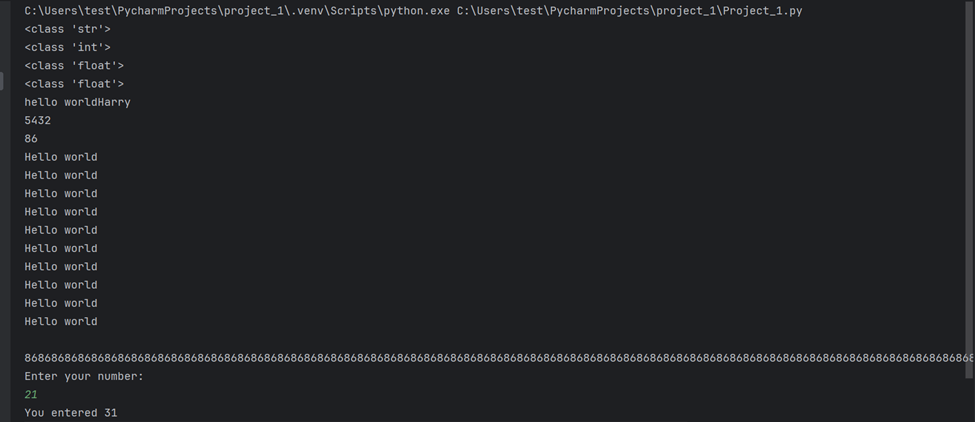

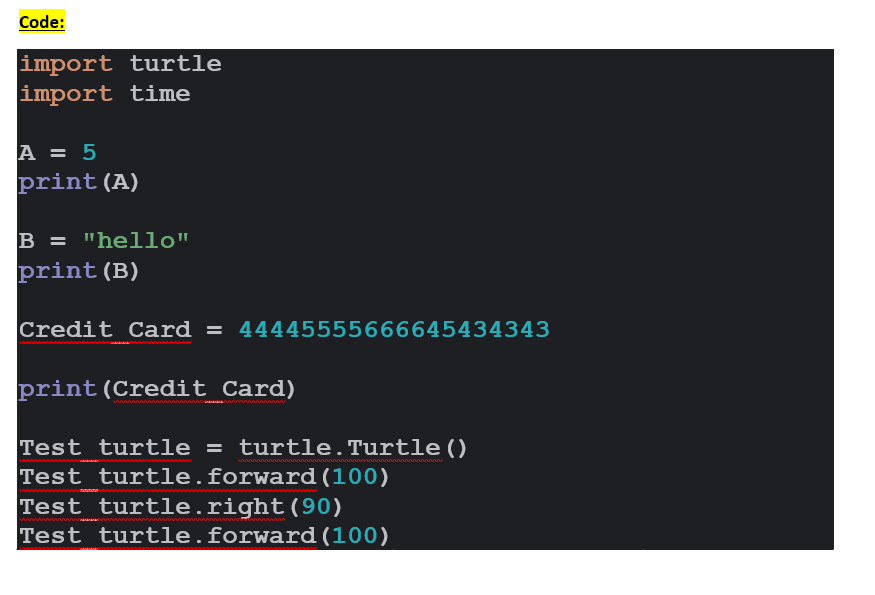
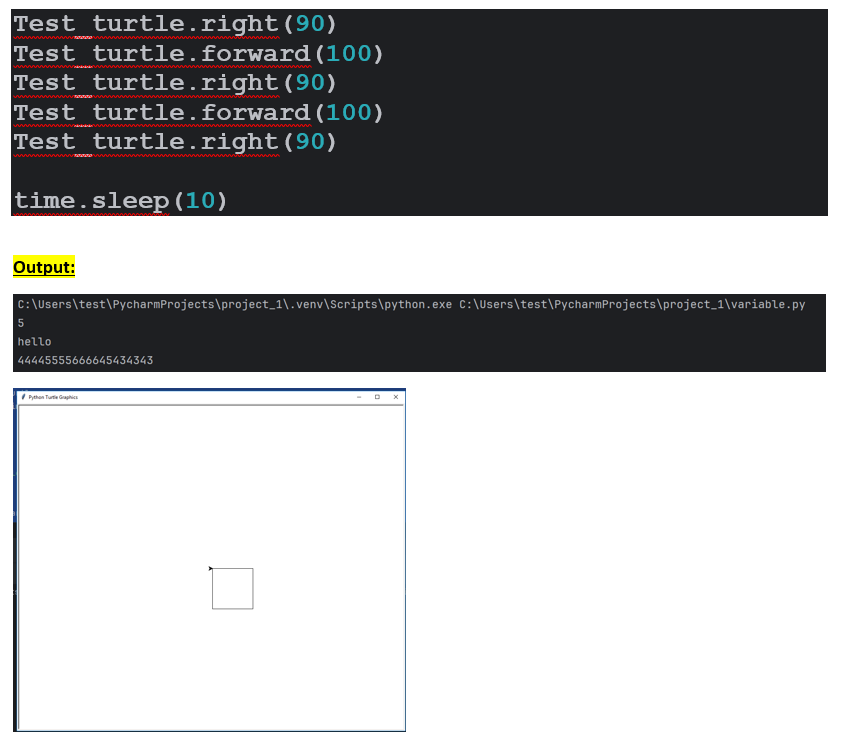